import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;是需要什么依赖
时间: 2024-08-15 16:09:11 浏览: 58
`ServiceImpl`通常是在MyBatis Plus框架中用于实现Service(服务层)接口的类模板。它依赖于以下几个核心组件:
1. **MyBatis Plus**:这是一个基于MyBatis的ORM(Object-Relational Mapping,对象关系映射)工具包,提供简化数据库操作的功能,如自动 CRUD(Create, Read, Update, Delete)等。
2. **Spring Framework**:如果在Spring Boot项目中使用,那么还会隐含地依赖Spring容器,因为它常用于依赖注入(DI)和服务注册与发现。
3. **Entity(实体类)**:`ServiceImpl`需要访问数据库表对应的实体类,以便将查询结果映射到Java对象。
4. **Mapper Interface(Mapper接口)**:Mapper接口定义了SQL操作,`ServiceImpl`会通过`@Autowired`注解注入相应的Mapper实现。
5. Spring Data JPA 或者 JDBC 驱动:如果你在使用JPA,那么还需要Spring Data JPA依赖;如果是原生的JDBC,就需要数据库连接池和JDBC驱动。
相关问题
package com.xinzhi.service.impl; import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; import com.xinzhi.mapper.HotelMapper; import com.xinzhi.pojo.Hotel; import com.xinzhi.service.IHotelService; import org.springframework.stereotype.Service; @Service public class HotelService extends ServiceImpl<HotelMapper, Hotel> implements IHotelService { }
根据你提供的代码,`HotelService` 类继承了 `ServiceImpl<HotelMapper, Hotel>`,并实现了 `IHotelService` 接口。根据代码,`HotelService` 类应该是一个有效的 Spring bean。
根据你的错误信息,问题似乎出现在 `baseMapper` 字段上。请确保以下几点:
1. `HotelMapper` 接口是有效的,并且与 `com.xinzhi.mapper` 包中的实现类相匹配。
2. `HotelMapper` 接口被正确地扫描并声明为一个 Spring bean。你可以在接口上添加 `@Repository` 注解来标记它。
3. 确保在 `HotelService` 类的 `baseMapper` 字段上使用了正确的注解。应该使用 `@Autowired` 注解进行自动装配。
如果你已经检查过以上几点,但问题仍然存在,可能是其他配置文件或组件引起的问题。如果你能提供更多的代码和配置细节,我可以更具体地帮助你解决问题。
package com.design.info.service; import com.baomidou.mybatisplus.core.toolkit.StringUtils; import com.baomidou.mybatisplus.core.toolkit.Wrappers; import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; import com.design.info.bean.Cate; import com.design.info.dao.CateMapper; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.List; @Service public class CateService extends ServiceImpl<CateMapper, Cate> { @Resource private CateMapper cateMapper; public List<Cate> listCate(Cate cate) { return cateMapper.selectList(Wrappers.<Cate>lambdaQuery() .like(StringUtils.isNotBlank(cate.getName()), Cate::getName, cate.getName()) .eq(cate.getActive() != null, Cate::getActive, cate.getActive()) .orderByAsc(Cate::getSort) ); } }
这段代码是一个使用了 MyBatis Plus 的 Service 层示例,继承了 `ServiceImpl<CateMapper, Cate>` 类,该类提供了一些常用的数据库操作方法的实现。
在该示例中,`CateService` 类使用了 `@Service` 注解来标识它是一个 Service 类,并注入了 `CateMapper` 对象来进行数据库操作。
其中,`listCate()` 方法用于查询符合条件的分类列表。在该方法中,使用了 MyBatis Plus 提供的 `Wrappers.<Cate>lambdaQuery()` 来创建查询条件构造器,使用了链式调用的方式进行条件拼接。
- `like(StringUtils.isNotBlank(cate.getName()), Cate::getName, cate.getName())`:当传入的 `cate` 对象的 `name` 字段不为空时,使用 `like` 条件进行模糊查询。
- `eq(cate.getActive() != null, Cate::getActive, cate.getActive())`:当传入的 `cate` 对象的 `active` 字段不为 null 时,使用 `eq` 条件进行等值查询。
- `orderByAsc(Cate::getSort)`:按照分类的 `sort` 字段进行升序排序。
最后,通过调用 `cateMapper.selectList()` 方法来执行查询操作,并返回查询结果列表。
希望对您有所帮助!如果您还有其他问题,请随时提问。
阅读全文
相关推荐
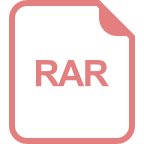
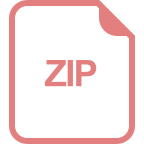

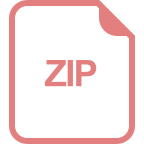
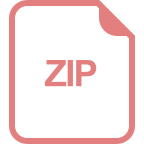











