题目14: 设采用拉链法构造散列表,并解决冲突,试设计算法实现散列表搜索和插入操作,给出c语言实现
时间: 2024-11-18 14:27:19 浏览: 16
题目14中的散列表(Hash Table),又称为哈希表,是一种通过哈希函数将键(Key)映射到数组索引来高效存储和查找数据的数据结构。拉链法(Chaining)是一种处理冲突(Collision, 当两个键被哈希函数映射到相同的槽位时)的常用方法,它在每个槽位上维护一个链表。
以下是使用拉链法在C语言中实现散列表搜索(Search)和插入(Insertion)的基本步骤:
**1. 定义散列表结构体**
```c
typedef struct Node {
void* data; // 存储的数据
struct Node* next; // 指向下一个节点的指针
} HashNode;
typedef struct HashTable {
int size; // 散列表大小
HashNode** table; // 用于存储元素的数组
} HashTable;
```
**2. 初始化散列表**
```c
HashTable* createHashTable(int size) {
HashTable* ht = (HashTable*)malloc(sizeof(HashTable));
ht->size = size;
ht->table = (HashNode**)calloc(size, sizeof(HashNode*));
for (int i = 0; i < size; i++) {
ht->table[i] = NULL;
}
return ht;
}
```
**3. 哈希函数**
这取决于具体的业务需求,这里假设简单的线性探测哈希函数(对于均匀分布的键,效果较好)
```c
unsigned int hashFunction(const char* key, int size) {
unsigned int hash = 5381; // 一种常用的简单哈希函数
while (*key) {
hash = ((hash << 5) + hash) + *key++;
}
return hash % size;
}
```
**4. 插入操作(Insert)**
```c
void insert(HashTable* ht, const char* key, void* value) {
int index = hashFunction(key, ht->size);
HashNode* newNode = (HashNode*)malloc(sizeof(HashNode));
newNode->data = value;
newNode->next = ht->table[index];
ht->table[index] = newNode;
// 如果冲突链表已满,则需要扩容
if (ht->table[index] != NULL && ht->table[index]->next == NULL) {
resizeHashTable(ht, 2 * ht->size);
}
}
// 扩容函数,这里省略
```
**5. 搜索操作(Search)**
```c
void* search(HashTable* ht, const char* key) {
int index = hashFunction(key, ht->size);
HashNode* current = ht->table[index];
while (current != NULL) {
if (!strcmp(current->data, key)) { // 使用strcmp比较键值是否相等
return current->data;
}
current = current->next;
}
return NULL; // 如果未找到,则返回NULL
}
```
以上代码仅为基本框架,实际应用中可能还需要考虑更复杂的哈希函数、负载因子以及链表过长时的优化处理。记得在不需要这些节点时释放内存,防止内存泄漏。
阅读全文
相关推荐
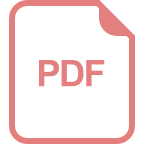
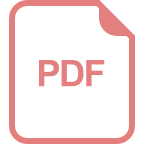
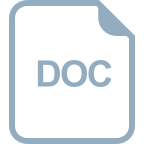
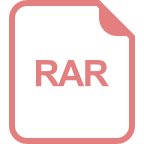
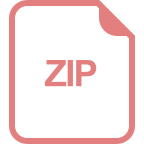
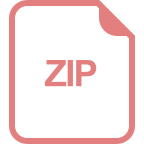
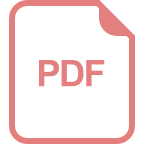
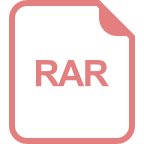
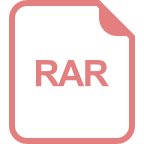
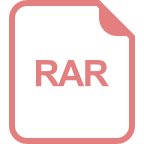
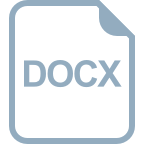
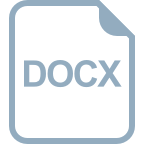
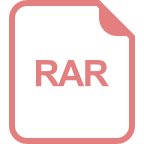
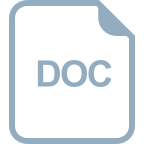
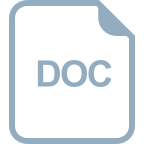
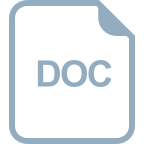
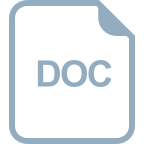