c获取当前文件夹下所有文件名
时间: 2023-09-28 14:01:56 浏览: 119
在C语言中,要获取当前文件夹下的所有文件名,可以使用系统库中的dirent.h头文件的相关函数。
首先,需要通过DIR指针打开当前文件夹。可以使用opendir函数来打开当前目录,并将返回的DIR指针赋值给相应的变量。例如:
DIR *dir;
dir = opendir(".");
接下来,可以使用readdir函数来读取当前目录中的文件和子目录,并通过一个循环将所有的文件名打印出来。例如:
struct dirent *entry;
while ((entry = readdir(dir)) != NULL) {
printf("%s\n", entry->d_name);
}
最后,记得在使用完DIR指针后关闭当前目录。可以使用closedir函数来关闭DIR指针。例如:
closedir(dir);
这样就完成了获取当前文件夹下所有文件名的操作。
请注意:上述代码只是一个简单的示例,如果需要更复杂的操作,比如获取特定文件类型或者遍历子目录等,可能需要更多的代码逻辑和处理。
相关问题
c语言获取文件夹下的所有文件名
在C语言中,可以使用以下代码来获取文件夹下的所有文件名:
```c
#include <dirent.h>
#include <stdio.h>
#include <string.h>
void getFilesInFolder(const char *folderPath) {
DIR *dir;
struct dirent *entry;
if ((dir = opendir(folderPath)) == NULL) {
printf("Can't open folder %s\n", folderPath);
return;
}
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue; // Skip current directory and parent directory
}
printf("%s/%s\n", folderPath, entry->d_name);
}
closedir(dir);
}
int main() {
const char *folderPath = "/home/xxx/images/";
getFilesInFolder(folderPath);
return 0;
}
```
这段代码使用`opendir`函数打开指定文件夹,然后使用`readdir`函数逐个读取文件夹中的文件名。通过判断文件名是否为`.`或`..`来排除当前目录和父目录。最后,将文件夹路径和文件名拼接打印出来。
写一个linux下用c++写的获取文件夹下所有文件名的代码
```
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <string.h>
int main(int argc, char *argv[]) {
DIR *dir; // 目录指针
struct dirent *entry; // 目录项指针
// 判断参数是否正确
if (argc != 2) {
fprintf(stderr, "Usage: %s <directory>\n", argv[0]);
exit(EXIT_FAILURE);
}
// 打开目录
if ((dir = opendir(argv[1])) == NULL) {
perror("opendir");
exit(EXIT_FAILURE);
}
// 遍历目录项并输出文件名
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue; // 跳过当前目录和父目录
}
printf("%s\n", entry->d_name);
}
// 关闭目录
closedir(dir);
return 0;
}
```
编译命令:
```
gcc -o get_filenames get_filenames.c
```
运行命令:
```
./get_filenames <directory>
```
阅读全文
相关推荐
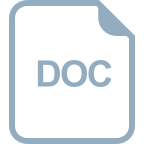
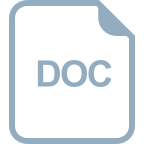
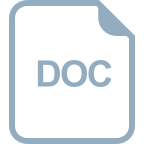
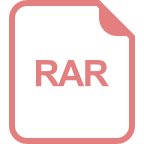
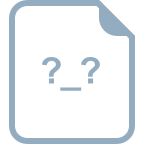
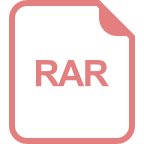
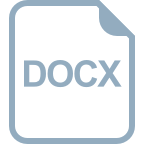
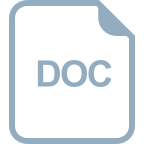


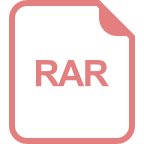
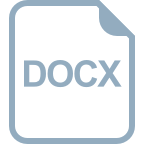
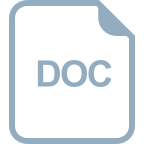


