如何处理数据库查询返回的"No Information Available"情况?
时间: 2024-10-24 08:08:33 浏览: 7
处理数据库查询返回"No Information Available"的情况通常需要考虑程序的健壮性和用户体验。以下是一些常见的做法:
1. **错误处理**:在代码中捕获异常,当查询结果为空时,可以抛出一个自定义的异常或者返回一个友好的错误消息给用户,说明数据不存在或暂无可用信息。
```python
try:
data = database.query(some_condition)
except NoInformationAvailableError:
message = "当前没有找到匹配的数据"
return message
```
2. **默认值或空值检查**:在获取数据后,先检查是否为空,如果是,则提供默认值、null值或者显示提示信息给用户。
```python
data = database.query(some_condition)
if not data:
data = get_default_value() or "暂时无相关信息"
```
3. **缓存策略**:如果频繁遇到无数据的情况,可以考虑将这类查询结果缓存起来,下次请求时直接从缓存查找,减少对数据库的压力。
4. **优化查询**:确认查询条件是否准确,并确保查询效率。如果查询结果总是空的,可能是查询逻辑有问题,需要调整SQL语句。
5. **反馈机制**:对于一些重要操作,如用户搜索,可以在返回结果之前提供一种反馈机制,让用户知道搜索正在进行中,而不仅仅是简单地告诉他们没有找到信息。
相关问题
C# 通过SQL数据库自动更新程序
要实现C#程序通过SQL数据库自动更新程序,您可以按照以下步骤进行操作:
1. 在SQL Server中创建一个表,用于存储程序版本号和程序的下载链接。表结构示例如下:
```
CREATE TABLE [dbo].[AppVersions](
[Version] [varchar](50) NOT NULL,
[DownloadUrl] [varchar](max) NOT NULL,
CONSTRAINT [PK_AppVersions] PRIMARY KEY CLUSTERED
(
[Version] ASC
) ON [PRIMARY]
) ON [PRIMARY] TEXTIMAGE_ON [PRIMARY]
```
2. 在C#程序中添加一个更新程序的功能,代码示例如下:
```
private void CheckUpdate()
{
string connectionString = "Data Source=yourServerName;Initial Catalog=yourDatabaseName;Integrated Security=True";
string query = "SELECT TOP 1 [Version], [DownloadUrl] FROM [dbo].[AppVersions] ORDER BY [Version] DESC";
using (SqlConnection connection = new SqlConnection(connectionString))
{
SqlCommand command = new SqlCommand(query, connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
if (reader.Read())
{
string latestVersion = reader.GetString(0);
string downloadUrl = reader.GetString(1);
if (latestVersion != Application.ProductVersion)
{
DialogResult result = MessageBox.Show("A new version is available. Do you want to download and install it now?", "Update Available", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
if (result == DialogResult.Yes)
{
WebClient webClient = new WebClient();
webClient.DownloadFile(downloadUrl, "update.exe");
Process.Start("update.exe");
Application.Exit();
}
}
else
{
MessageBox.Show("Your application is up-to-date.", "Update", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
else
{
MessageBox.Show("No updates available.", "Update", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
reader.Close();
}
}
```
3. 在程序启动时调用CheckUpdate()方法,检查是否有新版本可用。如果有,弹出提示框让用户选择是否下载并安装新版本。
需要注意的是,此方法只适用于Windows应用程序,如果您的程序是Web应用程序,则需要采用其他的更新方式。
阅读全文
相关推荐
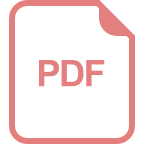
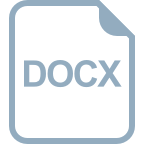
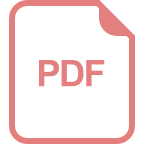
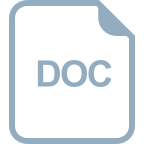
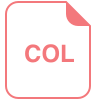
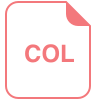
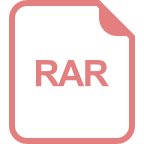
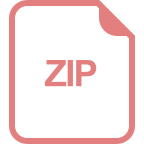
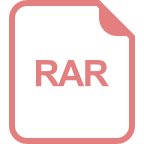
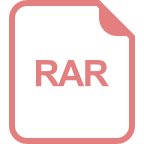
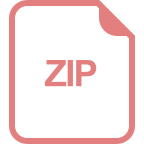
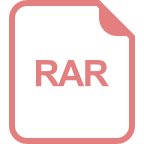
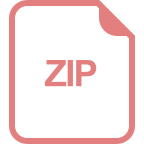
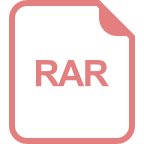