使用php实现这个代码
时间: 2024-12-16 21:19:56 浏览: 2
要实现“许愿墙”项目的核心功能,你需要按照以下步骤使用PHP编写代码:
### 1. 项目准备
#### 1.1 目录结构划分
项目的基本目录结构如下:
```
project/
├── common/
│ ├── function.php
│ └── init.php
├── css/
│ └── style.css
├── js/
│ └── common.js
├── view/
│ ├── common/
│ │ ├── add.html
│ │ ├── edit.html
│ │ └── password.html
│ └── index.html
├── index.php
└── save.php
```
#### 1.2 数据库设计
创建名为 `php_wish` 的数据库,并在其中创建 `wish` 表:
```sql
CREATE DATABASE php_wish;
USE php_wish;
CREATE TABLE wish (
id INT UNSIGNED PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(12) DEFAULT '' NOT NULL,
content VARCHAR(80) DEFAULT '' NOT NULL,
time INT UNSIGNED DEFAULT 0 NOT NULL,
color VARCHAR(10) DEFAULT '' NOT NULL,
password VARCHAR(6) DEFAULT '' NOT NULL
);
```
#### 1.3 连接数据库
在 `common/init.php` 中编写连接数据库的代码:
```php
<?php
// 设置时区
date_default_timezone_set('Asia/Shanghai');
// 数据库配置
$host = 'localhost';
$username = 'root';
$password = '';
$database = 'php_wish';
// 创建连接
$conn = new mysqli($host, $username, $password, $database);
// 检查连接
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
?>
```
#### 1.4 准备公共函数
在 `common/function.php` 中编写公共函数:
```php
<?php
/**
* 接收外部变量并进行过滤
*
* @param string $name 变量名称
* @param mixed $default 默认值
* @param string $method 方法(GET/POST)
* @param string $type 类型(s:字符串, d:整型, a:数组)
* @return mixed
*/
function input($name, $default = '', $method = 'GET', $type = 's') {
switch (strtoupper($method)) {
case 'GET':
$var = isset($_GET[$name]) ? $_GET[$name] : $default;
break;
case 'POST':
$var = isset($_POST[$name]) ? $_POST[$name] : $default;
break;
default:
return $default;
}
switch ($type) {
case 's': // 字符串
return filter_var($var, FILTER_SANITIZE_STRING);
case 'd': // 整型
return filter_var($var, FILTER_VALIDATE_INT);
case 'a': // 数组
if (!is_array($var)) {
return [];
}
return array_map('filter_var', $var, array_fill(0, count($var), FILTER_SANITIZE_STRING));
default:
return $default;
}
}
/**
* 格式化日期
*
* @param int $timestamp 时间戳
* @return string
*/
function format_date($timestamp) {
$time = time();
$diff = $time - $timestamp;
if ($diff < 60) { // 小于1分钟
return $diff . '秒前';
} elseif ($diff < 3600) { // 小于1小时
return floor($diff / 60) . '分钟前';
} elseif ($diff < 86400) { // 小于1天
return floor($diff / 3600) . '小时前';
} else { // 大于等于1天
return date('Y-m-d H:i:s', $timestamp);
}
}
?>
```
#### 1.5 引入公共文件
在 `index.php` 和 `save.php` 中引入公共文件:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
?>
```
### 2. 许愿墙展示
#### 2.1 查询所有愿望
在 `index.php` 中查询所有愿望:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
// 查询所有愿望
$sql = "SELECT id, name, content, time, color FROM wish";
$result = $conn->query($sql);
$wishes = [];
while ($row = $result->fetch_assoc()) {
$row['time'] = format_date($row['time']);
$wishes[] = $row;
}
// 关闭数据库连接
$conn->close();
?>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<title>许愿墙</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div id="container">
<?php foreach ($wishes as $wish): ?>
<div class="wish <?php echo htmlspecialchars($wish['color']); ?>">
<span><?php echo htmlspecialchars($wish['name']); ?></span>
<p><?php echo htmlspecialchars($wish['content']); ?></p>
<small><?php echo $wish['time']; ?></small>
<a href="index.php?action=edit&id=<?php echo $wish['id']; ?>">修改</a>
<a href="index.php?action=delete&id=<?php echo $wish['id']; ?>">删除</a>
</div>
<?php endforeach; ?>
</div>
<button onclick="showAddForm()">我要许愿</button>
<!-- 添加愿望表单 -->
<div id="add-form" style="display:none;">
<form action="save.php" method="post">
<label for="name">姓名:</label>
<input type="text" name="name" required><br>
<label for="content">愿望:</label>
<textarea name="content" required></textarea><br>
<label for="color">颜色:</label>
<select name="color">
<option value="red">红色</option>
<option value="blue">蓝色</option>
<option value="green">绿色</option>
</select><br>
<label for="password">密码:</label>
<input type="password" name="password"><br>
<button type="submit">提交</button>
</form>
</div>
<script src="js/common.js"></script>
</body>
</html>
```
### 3. 发表愿望
#### 3.1 创建发表愿望表单
在 `view/common/add.html` 中创建表单:
```html
<form action="save.php" method="post">
<label for="name">姓名:</label>
<input type="text" name="name" required><br>
<label for="content">愿望:</label>
<textarea name="content" required></textarea><br>
<label for="color">颜色:</label>
<select name="color">
<option value="red">红色</option>
<option value="blue">蓝色</option>
<option value="green">绿色</option>
</select><br>
<label for="password">密码:</label>
<input type="password" name="password"><br>
<button type="submit">提交</button>
</form>
```
#### 3.2 接收表单并进行过滤
在 `save.php` 中接收表单并进行过滤:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
$name = input('name', '', 'POST', 's');
$content = input('content', '', 'POST', 's');
$color = input('color', 'red', 'POST', 's');
$password = input('password', '', 'POST', 's');
// 验证输入长度
$name = mb_strimwidth($name, 0, 12, '');
$content = mb_strimwidth($content, 0, 80, '');
// 插入数据
$stmt = $conn->prepare("INSERT INTO wish (name, content, time, color, password) VALUES (?, ?, ?, ?, ?)");
$time = time();
$stmt->bind_param("ssiss", $name, $content, $time, $color, $password);
if ($stmt->execute()) {
header("Location: index.php");
exit;
} else {
echo "Error: " . $stmt->error;
}
$stmt->close();
$conn->close();
?>
```
### 4. 修改愿望
#### 4.1 验证保护密码
在 `index.php` 中验证保护密码:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
$action = input('action', '', 'GET', 's');
$id = input('id', 0, 'GET', 'd');
if ($action == 'edit' && $id > 0) {
$sql = "SELECT * FROM wish WHERE id = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("i", $id);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows > 0) {
$wish = $result->fetch_assoc();
$stmt->close();
if (isset($_POST['password'])) {
$password = input('password', '', 'POST', 's');
if ($password == $wish['password']) {
// 密码验证成功,显示修改表单
include 'view/common/edit.html';
exit;
} else {
echo "密码错误,请重新输入。";
}
} else {
// 显示输入密码的表单
include 'view/common/password.html';
exit;
}
} else {
echo "愿望不存在。";
}
}
?>
```
#### 4.2 接收修改愿望的表单
在 `save.php` 中接收修改愿望的表单:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
$id = input('id', 0, 'POST', 'd');
$name = input('name', '', 'POST', 's');
$content = input('content', '', 'POST', 's');
$color = input('color', 'red', 'POST', 's');
$password = input('password', '', 'POST', 's');
if ($id > 0) {
// 验证输入长度
$name = mb_strimwidth($name, 0, 12, '');
$content = mb_strimwidth($content, 0, 80, '');
// 更新数据
$stmt = $conn->prepare("UPDATE wish SET name = ?, content = ?, color = ?, password = ? WHERE id = ?");
$stmt->bind_param("sssii", $name, $content, $color, $password, $id);
if ($stmt->execute()) {
header("Location: index.php");
exit;
} else {
echo "Error: " . $stmt->error;
}
} else {
// 执行添加操作
$stmt = $conn->prepare("INSERT INTO wish (name, content, time, color, password) VALUES (?, ?, ?, ?, ?)");
$time = time();
$stmt->bind_param("ssiss", $name, $content, $time, $color, $password);
if ($stmt->execute()) {
header("Location: index.php");
exit;
} else {
echo "Error: " . $stmt->error;
}
}
$stmt->close();
$conn->close();
?>
```
### 5. 删除愿望
在 `index.php` 中实现删除愿望的功能:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
$action = input('action', '', 'GET', 's');
$id = input('id', 0, 'GET', 'd');
if ($action == 'delete' && $id > 0) {
$sql = "SELECT * FROM wish WHERE id = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("i", $id);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows > 0) {
$wish = $result->fetch_assoc();
$stmt->close();
if (isset($_POST['password'])) {
$password = input('password', '', 'POST', 's');
if ($password == $wish['password']) {
// 密码验证成功,删除愿望
$stmt = $conn->prepare("DELETE FROM wish WHERE id = ?");
$stmt->bind_param("i", $id);
if ($stmt->execute()) {
header("Location: index.php");
exit;
} else {
echo "Error: " . $stmt->error;
}
} else {
echo "密码错误,请重新输入。";
}
} else {
// 显示输入密码的表单
include 'view/common/password.html';
exit;
}
} else {
echo "愿望不存在。";
}
}
?>
```
### 6. 分页查询
在 `index.php` 中实现分页查询:
```php
<?php
require_once 'common/init.php';
require_once 'common/function.php';
$page = input('page', 1, 'GET', 'd');
$size = 10;
// 获取总记录数
$sql = "SELECT COUNT(*) AS total FROM wish";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
$total = $row['total'];
$total_pages = ceil($total / $size);
// 分页查询
$start = ($page - 1) * $size;
$sql = "SELECT id, name, content, time, color FROM wish ORDER BY id DESC LIMIT ?, ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("ii", $start, $size);
$stmt->execute();
$result = $stmt->get_result();
$wishes = [];
while ($row = $result->fetch_assoc()) {
$row['time'] = format_date($row['time']);
$wishes[] = $row;
}
// 关闭数据库连接
$stmt->close();
$conn->close();
?>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<title>许愿墙</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div id="container">
<?php foreach ($wishes as $wish): ?>
<div class="wish <?php echo htmlspecialchars($wish['color']); ?>">
<span><?php echo htmlspecialchars($wish['name']); ?></span>
<p><?php echo htmlspecialchars($wish['content']); ?></p>
<small><?php echo $wish['time']; ?></small>
<a href="index.php?action=edit&id=<?php echo $wish['id']; ?>">修改</a>
<a href="index.php?action=delete&id=<?php echo $wish['id']; ?>">删除</a>
</div>
<?php endforeach; ?>
</div>
<nav>
<a href="index.php?page=1">首页</a>
<a href="index.php?page=<?php echo max(1, $page - 1); ?>">上一页</a>
<a href="index.php?page=<?php echo min($total_pages, $page + 1); ?>">下一页</a>
<a href="index.php?page=<?php echo $total_pages; ?>">尾页</a>
</nav>
<button onclick="showAddForm()">我要许愿</button>
<!-- 添加愿望表单 -->
<div id="add-form" style="display:none;">
<form action="save.php" method="post">
<label for="name">姓名:</label>
<input type="text" name="name" required><br>
<label for="content">愿望:</label>
<textarea name="content" required></textarea><br>
<label for="color">颜色:</label>
<select name="color">
<option value="red">红色</option>
<option value="blue">蓝色</option>
<option value="green">绿色</option>
</select><br>
<label for="password">密码:</label>
<input type="password" name="password"><br>
<button type="submit">提交</button>
</form>
</div>
<script src="js/common.js"></script>
</body>
</html>
```
### 7. 其他文件
- **CSS文件**:`css/style.css`
- **JavaScript文件**:`js/common.js`
- **HTML模板文件**:`view/common/add.html`, `view/common/edit.html`, `view/common/password.html`, `view/index.html`
这些文件的具体内容可以根据项目需求进行调整和完善。希望这些代码能帮助你顺利完成“许愿墙”项目!
阅读全文
相关推荐
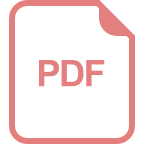
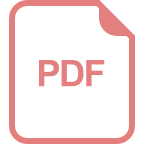
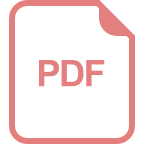
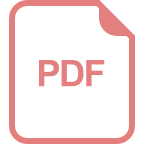
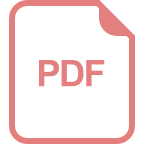
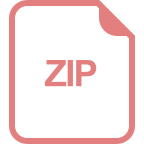
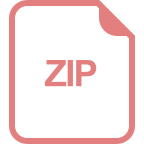
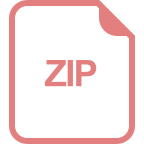
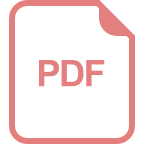
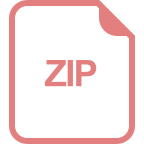
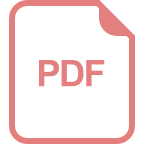
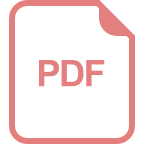
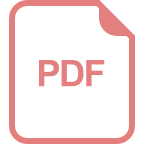
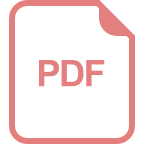
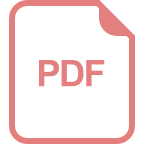