在C#中,如何利用HttpListener类构建一个简易的HTTP服务器,并处理HTTP请求?请提供详细的步骤和代码示例。
时间: 2024-11-18 20:22:46 浏览: 2
要使用C#中的HttpListener类构建一个简易的HTTP服务器,首先需要了解HttpListener的用途及其工作原理。HttpListener是.NET Framework提供的一个类,允许开发者在应用程序中监听和响应HTTP请求,它作为一个内置的HTTP服务器,非常适合用于创建轻量级的Web服务或者用于教学目的。以下是构建简易HTTP服务器的步骤和代码示例:
参考资源链接:[C#简易HTTP服务器:HttpListener与自定义处理](https://wenku.csdn.net/doc/6401ababcce7214c316e9128?spm=1055.2569.3001.10343)
1. 引入必要的命名空间:
```csharp
using System;
***;
***.HttpListener;
using System.Threading;
using System.IO;
```
2. 初始化HttpListener对象,并指定监听的URL前缀:
```csharp
string prefix =
参考资源链接:[C#简易HTTP服务器:HttpListener与自定义处理](https://wenku.csdn.net/doc/6401ababcce7214c316e9128?spm=1055.2569.3001.10343)
相关问题
如何利用C#的HttpListener类构建一个简易的HTTP服务器,并高效处理HTTP请求以提高性能?
在C#中,使用HttpListener类构建简易HTTP服务器时,可以通过优化监听机制和响应处理来提升服务器性能。以下是详细步骤和代码示例:
参考资源链接:[C#简易HTTP服务器:HttpListener与自定义处理](https://wenku.csdn.net/doc/6401ababcce7214c316e9128?spm=1055.2569.3001.10343)
1. 初始化HttpListener类实例,并注册前缀。例如,监听本地地址和8080端口:httpListener.Prefixes.Add(
参考资源链接:[C#简易HTTP服务器:HttpListener与自定义处理](https://wenku.csdn.net/doc/6401ababcce7214c316e9128?spm=1055.2569.3001.10343)
c# 创建简单的HttpListener服务器
下面是一个使用C#创建简单的HttpListener服务器的示例代码:
```csharp
using System;
using System.Net;
using System.Text;
public class SimpleHttpServer
{
public static void Main(string[] args)
{
// 设置监听的地址和端口号
string url = "http://localhost:8080/";
// 创建HttpListener对象
HttpListener listener = new HttpListener();
// 添加要监听的地址
listener.Prefixes.Add(url);
// 开始监听
listener.Start();
Console.WriteLine("Server is running...");
while (true)
{
// 等待客户端请求
HttpListenerContext context = listener.GetContext();
// 获取请求对象
HttpListenerRequest request = context.Request;
// 获取请求的HTTP方法(GET, POST等)
string httpMethod = request.HttpMethod;
// 获取请求的URL
string urlRequest = request.Url.ToString();
// 获取请求的内容
string requestBody = new System.IO.StreamReader(request.InputStream, request.ContentEncoding).ReadToEnd();
// 构建响应内容
string responseBody = "<html><body><h1>Hello, World!</h1></body></html>";
// 将响应内容转换为字节数组
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
// 设置响应状态码
context.Response.StatusCode = 200;
// 设置响应内容长度
context.Response.ContentLength64 = buffer.Length;
// 发送响应内容
context.Response.OutputStream.Write(buffer, 0, buffer.Length);
// 关闭响应
context.Response.OutputStream.Close();
}
}
}
```
这个例子创建一个HttpListener对象,并设置要监听的地址和端口号。然后使用while循环等待客户端请求。当有客户端请求到达时,它获取请求对象,并从请求对象中获取HTTP方法,URL和内容。然后构建响应内容,并将其发送回客户端。
阅读全文
相关推荐
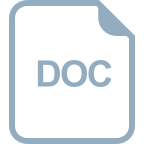
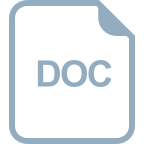
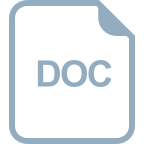
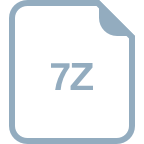
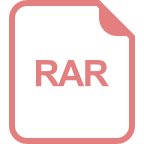
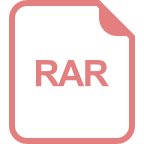
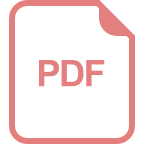
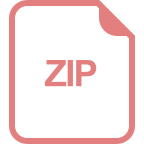
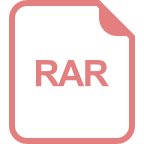
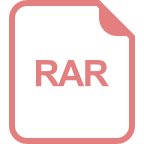
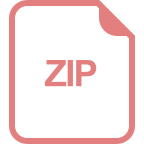
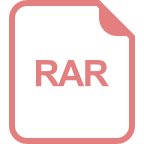
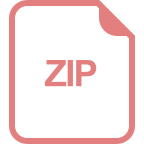
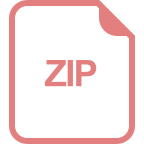
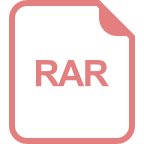
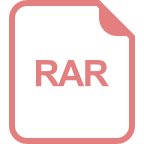
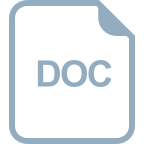