蒙特卡洛树搜索算法五子棋
时间: 2024-12-26 19:15:36 浏览: 3
### Monte Carlo Tree Search Algorithm Implementation for Gomoku
Monte Carlo Tree Search (MCTS) is a heuristic search algorithm often used in artificial intelligence applications, especially game playing. In the context of Gomoku or Five-in-a-Row, MCTS can be implemented to create an intelligent opponent that makes decisions based on simulations and evaluations.
#### Key Components of MCTS
The core components include selection, expansion, simulation, and backpropagation:
- **Selection**: Traverse nodes from root until reaching leaf node.
- **Expansion**: Add one or more child nodes to the leaf node selected during Selection phase.
- **Simulation**: Run random playouts starting at expanded node.
- **Backpropagation**: Update statistics up through tree hierarchy after Simulation completes.
```python
import numpy as np
from copy import deepcopy
class TreeNode(object):
def __init__(self, parent=None, prior_p=1.0):
self._parent = parent
self._children = {}
self._n_visits = 0
self._Q = 0
self._u = 0
self._P = prior_p
def select_action(node, c_param=5):
"""Select action with highest upper confidence bound."""
best_value = float("-inf")
best_actions = []
for action, child_node in node._children.items():
value = child_node.get_value(c_param)
if value > best_value:
best_value = value
best_actions = [action]
elif value == best_value:
best_actions.append(action)
return np.random.choice(best_actions)
def expand_and_evaluate(board_state, player_turn):
available_moves = board_state.get_legal_moves()
move = np.random.choice(available_moves)
next_board_state = deepcopy(board_state).make_move(move, player_turn)
new_node = TreeNode(parent=current_node)
current_node._children[move] = new_node
winner = next_board_state.check_winner()
if winner != None:
reward = 1 if winner == player_turn else -1
else:
reward = simulate_random_playout(deepcopy(next_board_state), player_turn)
update_tree(current_node, reward)
def mcts_search(root_state, n_simulations=1000):
root_node = TreeNode(None, 1.0)
for _ in range(n_simulations):
node = root_node
while not node.is_leaf():
node = select_child_with_highest_uct(node)
if node.board_state.game_over():
continue
expand_and_evaluate(node.board_state, node.player_to_act())
most_visited_nodes = sorted(
[(child_node.n_visits, action) for action,
child_node in root_node.children.items()], reverse=True)[:1]
_, best_action = most_visited_nodes[0]
return best_action
```
This code snippet provides a basic framework for implementing MCTS specifically tailored towards games like Gomoku where players aim to align five stones consecutively either horizontally, vertically, or diagonally before their opponents do so[^1].
阅读全文
相关推荐
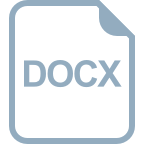
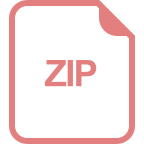
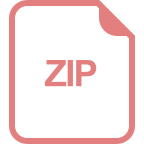
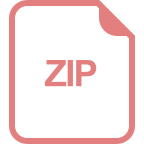
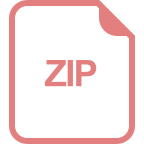
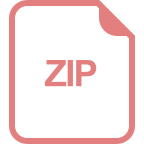
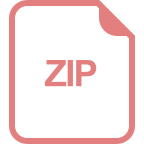
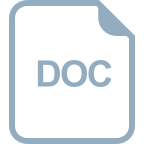
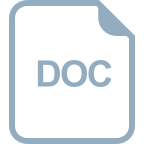
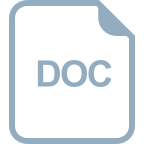
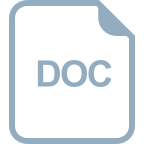
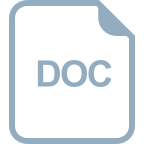
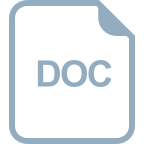
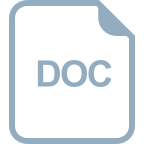


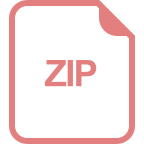