使用一个弹窗用于监听确认下拉框的@change事件在手机实机上是否被正确触发。
时间: 2024-11-29 08:37:00 浏览: 6
为了验证在手机实机上是否正确触发了下拉框的 `@change` 事件,可以通过以下步骤实现:
1. **添加弹窗组件**:在现有代码中添加一个新的弹窗组件,用于显示 `@change` 事件的触发情况。
2. **修改下拉框的 `@change` 事件处理器**:在现有的 `@change` 事件处理器中调用弹窗组件的显示方法,并传递相关信息。
以下是具体的实现步骤:
### 1. 添加弹窗组件
在现有模板中添加一个新的弹窗组件:
```html
<!-- 弹窗组件 -->
<view v-if="isChangeModalVisible" class="modal">
<view class="modal-content">
<h3>下拉框变更通知</h3>
<p>选择的区域: {{ selectedArea }}</p>
<button @click="closeChangeModal" style="width: 100px; height: 50px; font-size: 20px;">关闭</button>
</view>
</view>
```
### 2. 修改数据模型和方法
在 `data` 中添加新的布尔值变量 `isChangeModalVisible`,并在 `methods` 中添加相应的显示和关闭弹窗的方法:
```javascript
data() {
return {
// 其他数据...
isChangeModalVisible: false,
};
},
methods: {
// 其他方法...
handleSelectChange(event) {
const selectedValue = event.target.value;
this.selectedArea = selectedValue;
this.filterTab2Data();
this.showChangeModal(); // 显示弹窗
},
showChangeModal() {
this.isChangeModalVisible = true;
},
closeChangeModal() {
this.isChangeModalVisible = false;
}
}
```
### 3. 更新下拉框的 `@change` 事件
确保下拉框的 `@change` 事件绑定到 `handleSelectChange` 方法:
```html
<select v-model="selectedArea" @change="handleSelectChange" class="area-select">
<option value="">请选择检查区域</option>
<option v-for="area in areasTab2" :value="area" :key="area">{{ area }}</option>
</select>
```
### 完整代码示例
以下是完整的代码示例,包括新增的弹窗组件和相关的数据及方法:
```html
<template>
<view class="container">
<!-- Tab 切换按钮 -->
<view class="tab-bar">
<view class="tab-item" @click="currentTab = 'tab1'" :class="{ active: currentTab === 'tab1' }">日常检查</view>
<view class="tab-item" @click="currentTab = 'tab2'" :class="{ active: currentTab === 'tab2' }">专项检查</view>
</view>
<label></label>
<text>主任务名称:{{ taskId }}</text>
<!-- Tab 内容区域 -->
<view class="tab-content">
<view v-if="currentTab === 'tab1'" class="tab-page">
<select v-model="selectedArea" @change="filterTab1Data" class="area-select">
<option value="">请选择检查区域</option>
<option v-for="area in areasTab1" :value="area" :key="area">{{ area }}</option>
</select>
<view class="filter-bar"></view>
<view class="text-wrapper">
<view v-for="(item, index) in tab1Data.rows" :key="index" class="text-item">
<span class="input-prefix">检查内容: </span>
<input type="text" readonly v-model="item.Main_task_Nopri" class="input-field" />
<br />
<span class="input-prefix">危险源: </span>
<input type="text" readonly v-model="item.dangerous" class="input-field" />
<br />
<span class="input-prefix">工程技术措施: </span>
<input type="text" readonly v-model="item.Engineering_measures" class="input-field" />
<br />
<span class="input-prefix">管理措施: </span>
<input type="text" readonly v-model="item.management_measure" class="input-field" />
<br />
<select v-model="item.complianceStatus" @change="updateComplianceStatus(item)" class="compliance-status-select">
<option value="符合">符合</option>
<option value="不符合">不符合</option>
</select>
<br />
<button v-if="item.complianceStatus === '不符合'" @click="showDetail(item)">录入隐患</button>
</view>
</view>
</view>
<view v-if="currentTab === 'tab2'" class="tab-page">
<select v-model="selectedArea" @change="handleSelectChange" class="area-select">
<option value="">请选择检查区域</option>
<option v-for="area in areasTab2" :value="area" :key="area">{{ area }}</option>
</select>
<view class="text-wrapper">
<view v-for="(item, index) in anotherData.rows" :key="index" class="text-item">
<span class="input-prefix">检查项目: </span>
<input type="text" readonly v-model="item.check_pro" class="input-field" />
<br />
<span class="input-prefix">检查区域: </span>
<input type="text" readonly v-model="item.AREA_NAME" class="input-field" />
<br />
<span class="input-prefix">检查标准:</span>
<input type="text" readonly v-model="item.check_sta" @click="showCheckStandardModal(item.check_sta)" />
<br />
<u-form-item class="picupload" label="检查人签字:" prop="upload" labelWidth=80px>
<u-upload width="80" height="40" maxCount="6" @on-success="uploadUpload" @on-remove="delUpload" :action="actionUrl" v-model="upload"></u-upload>
</u-form-item>
<u-form-item class="picupload1" label="被检查区域负责人签字:" prop="upload" labelWidth=80px>
<div style="display:flex; justify-content-between;">
<u-upload width="80" height="40" maxCount="6" @on-success="uploadUpload" @on-remove="delUpload" :action="actionUrl" v-model="upload"></u-upload>
<button type="button" class="btn-add-file" @click="handleAddFile">点击进入签名</button>
</div>
</u-form-item>
<select v-model="item.complianceStatus" @change="updateComplianceStatus(item)" class="compliance-status-select">
<option value="符合">符合</option>
<option value="不符合">不符合</option>
</select>
<br />
<button v-if="item.complianceStatus === '不符合'" @click="showDetail(item)">录入隐患</button>
</view>
</view>
</view>
</view>
<!-- 弹窗组件 -->
<view v-if="isModalVisible" class="modal">
<view class="modal-content">
<h3>详细信息</h3>
<form @submit.prevent="updateItem">
<u-form-item class="picupload" label="隐患图片" prop="upload" labelWidth=80px>
<u-upload width="80" height="40" maxCount="6" @on-success="uploadUpload" @on-remove="delUpload" :action="actionUrl" v-model="upload"></u-upload>
</u-form-item>
<div>
<label>隐患描述:</label>
<input type="text" id="DANGER_DESC" v-model="selectedItem.DANGER_DESC" />
</div>
<button type="submit" style="width: 100px; height: 50px; font-size: 20px;">保存</button>
<button @click="closeModal" style="width: 100px; height: 50px; font-size: 20px;">关闭</button>
</form>
</view>
</view>
<view v-if="isAnotherModalVisible" class="modal">
<view class="modal-content">
<h3>检查标准详情</h3>
<div>
<p>{{ selectedItemForModal.check_sta }}</p>
</div>
<button @click="closeModal" style="width: 100px; height: 50px; font-size: 20px;">关闭</button>
</view>
</view>
<view v-if="isChangeModalVisible" class="modal">
<view class="modal-content">
<h3>下拉框变更通知</h3>
<p>选择的区域: {{ selectedArea }}</p>
<button @click="closeChangeModal" style="width: 100px; height: 50px; font-size: 20px;">关闭</button>
</view>
</view>
</view>
</template>
<script>
import request from '@/utils/request';
import { getToken } from '@/utils/auth';
export default {
data() {
return {
currentTab: 'tab1',
tab1Data: { status: null, rows: [], originalRows: [] },
taskId: '',
anotherData: { status: null, rows: [], originalRows: [] },
isModalVisible: false,
isAnotherModalVisible: false,
isChangeModalVisible: false,
selectedItem: { name: '', complianceStatus: '符合', hazardDescription: '' },
selectedArea: null,
areasTab1: [],
areasTab2: [],
selectedItemForModal: null,
upload: [],
actionUrl: ''
};
},
watch: {
selectedArea(newVal) {
if (this.currentTab === 'tab1') {
this.filterTab1Data();
} else if (this.currentTab === 'tab2') {
this.filterTab2Data();
}
}
},
methods: {
fetchTab1Data() {
request({
url: '/api/check_carryout_record_daily/getPageData',
method: 'POST',
header: { 'content-type': 'application/json', 'Authorization': `Bearer ${getToken()}` },
data: {},
dataType: 'json'
}).then(response => {
this.tab1Data.originalRows = response.rows.map(item => ({ ...item, complianceStatus: '符合' }));
this.filterTab1Data();
this.areasTab1 = [...new Set(response.rows.map(item => item.AREA_NAME))];
}).catch(error => {
console.error(error);
uni.showToast({ title: '请求失败', icon: 'none' });
});
},
fetchTab2Data() {
request({
url: '/api/check_carryout_record/getPageData',
method: 'POST',
header: { 'content-type': 'application/json', 'Authorization': `Bearer ${getToken()}` },
dataType: 'json'
}).then(response => {
this.anotherData.originalRows = response.rows.map(item => ({ ...item, complianceStatus: '符合' }));
this.filterTab2Data();
this.areasTab2 = [...new Set(response.rows.map(item => item.AREA_NAME))];
}).catch(error => {
console.error(error);
uni.showToast({ title: '请求失败', icon: 'none' });
});
},
showCheckStandardModal(checkSta) {
this.selectedItem = { checkSta };
this.isAnotherModalVisible = true;
},
handleSelectChange(event) {
const selectedValue = event.target.value;
this.selectedArea = selectedValue;
this.filterTab2Data();
this.showChangeModal(); // 显示弹窗
},
filterTab1Data() {
const taskId = String(this.taskId);
this.tab1Data.rows = this.tab1Data.originalRows.filter(item => {
return String(item.Main_task_Nopri) === taskId && (!this.selectedArea || item.AREA_NAME === this.selectedArea);
});
},
filterTab2Data() {
const taskId = String(this.taskId);
this.anotherData.rows = this.anotherData.originalRows.filter(item => {
return String(item.Main_task_Nopri) === taskId && (!this.selectedArea || item.AREA_NAME === this.selectedArea);
});
},
showDetail(item) {
this.selectedItem = { ...item };
this.isModalVisible = true;
},
closeModal() {
this.isModalVisible = false;
this.isAnotherModalVisible = false;
},
showChangeModal() {
this.isChangeModalVisible = true;
},
closeChangeModal() {
this.isChangeModalVisible = false;
},
updateItem() {
request({
url: '/api/update_item',
method: 'POST',
header: { 'content-type': 'application/json', 'Authorization': `Bearer ${getToken()}` },
data: this.selectedItem,
dataType: 'json'
}).then(response => {
this.closeModal();
this.fetchTab1Data();
uni.showToast({ title: '更新成功', icon: 'success' });
}).catch(error => {
console.error(error);
uni.showToast({ title: '更新失败', icon: 'none' });
});
},
updateComplianceStatus(item) {
// 这里可以添加额外的逻辑来处理合规状态变化,如果需要
},
onLoad(options) {
if (options.id) {
this.taskId = decodeURIComponent(options.id);
}
this.fetchTab1Data();
this.fetchTab2Data();
},
mounted() {}
}
};
</script>
<style lang="scss">
.container {
padding: 10px;
background-color: #f9f9f9;
}
.compliance-status-select {
width: 300px;
height: 25px;
}
.input-prefix {
display: inline-block;
vertical-align: middle;
width: 105px;
padding: 0px;
background-color: #ffffff;
text-align: left;
font-size: 16px;
}
.input-field {
border: 1px solid #ccc;
border-radius: 4px;
height: 30px;
margin-bottom: -20px;
}
.text-value {
display: inline-block;
vertical-align: middle;
width: calc(100% - 110px);
padding: 10px;
border: none;
border-radius: 4px;
margin-left: 15px;
font-size: 16px;
}
.tab-bar {
display: flex;
justify-content: space-around;
background-color: #f1f1f1;
padding: 10px 0;
}
.tab-item {
flex: 1;
text-align: center;
padding: 10px 0;
cursor: pointer;
}
.tab-item.active {
color: #409EFF;
border-bottom: 2px solid #409EFF;
}
.tab-content {
margin-top: 10px;
}
.tab-page {
padding: 12px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.text-item {
margin-bottom: 30px;
padding: 10px;
border: 1px solid #ddd;
border-radius: 4px;
.input-prefix + .input-field,
.input-prefix + select,
.input-prefix + button {
margin-top: 5px;
}
}
.modal {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
align-items: center;
justify-content: center;
}
.modal-content {
background-color: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.3);
max-width: 600px;
width: 100%;
}
.modal-content h3 {
margin-top: 0;
}
.modal-content label {
display: block;
margin-bottom: 5px;
}
.modal-content input {
display: block;
width: 100%;
height: 60px;
margin-bottom: 40px;
padding: 5px;
border: 1px solid #ccc;
border-radius: 4px;
}
.modal-content button {
margin-right: 20px;
border: none;
border-radius: 4px;
cursor: pointer;
padding: 0px 0px;
font-size: 16px;
}
.modal-content button[type="submit"] {
background-color: #4CAF50;
color: white;
}
.modal-content button[type="submit"]:hover {
background-color: #45a049;
}
.modal-content button[type="button"] {
background-color:
阅读全文
相关推荐
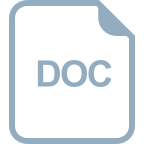
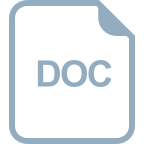
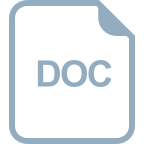

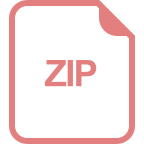
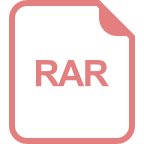
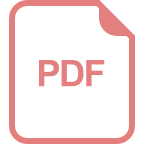
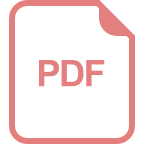










