Vue -- 如何实现页面每隔一段时间自动刷新
时间: 2024-09-09 22:03:32 浏览: 55
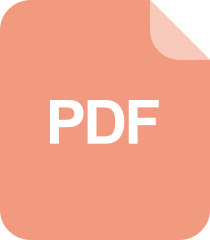
vue实现页面滚动到底部刷新
在Vue.js中,要实现页面每隔一段时间自动刷新,通常会利用`Intersection Observer API`或者结合定时器`setTimeout`函数。这里提供两种常见的方法:
1. **使用Intersection Observer**:
- 首先,安装`vue-popper`等库提供的`@popperjs/core`,因为它包含了一个简单的计时器组件`v-popover`,可以配合使用。
```javascript
import { Popper } from '@popperjs/core';
import { VPopover } from 'vue-popper';
export default {
data() {
return {
refreshInterval: null,
};
},
methods: {
startAutoRefresh() {
const observer = new IntersectionObserver((entries) => {
if (entries[0].isIntersecting) {
this.refresh();
}
});
observer.observe(document.querySelector('your-target-element')); // 替换为你想要观察的目标元素
// 每隔指定时间(例如5秒)检查一次目标元素是否可见
this.refreshInterval = setInterval(() => observer.check(), 5000);
},
stopAutoRefresh() {
clearInterval(this.refreshInterval);
},
refresh() {
// 当目标元素进入视野时,执行刷新操作,如路由跳转、数据更新等
this.$router.push('/refresh-page'); // 或者this.$set(this, 'data', ...); 等
},
},
mounted() {
this.startAutoRefresh();
},
beforeDestroy() {
this.stopAutoRefresh();
},
};
```
2. **使用setTimeout和clearTimeout**:
如果没有第三方库支持,可以直接使用JavaScript的`setTimeout`和`clearTimeout`来设置定时任务。
```javascript
data() {
return {
autoRefreshTimeoutId: null,
};
},
methods: {
startAutoRefresh() {
this.autoRefreshTimeoutId = setTimeout(() => {
this.refresh();
this.startAutoRefresh(); // 递归调用直到停止
}, 5000); // 设定刷新间隔,单位毫秒
},
stopAutoRefresh() {
clearTimeout(this.autoRefreshTimeoutId);
},
refresh() {
// 页面刷新内容,例如axios请求数据
axios.get('/refresh-url')
.then(response => {
// 更新视图
})
.finally(() => {
// 清除定时任务后开始下一轮刷新
this.startAutoRefresh();
});
},
},
mounted() {
this.startAutoRefresh();
},
beforeDestroy() {
this.stopAutoRefresh();
},
```
记得替换上述代码中的`your-target-element`和`/refresh-page`为实际的元素选择器和刷新路径。在适当的时候调用`stopAutoRefresh`方法来停止刷新。
阅读全文
相关推荐
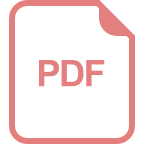
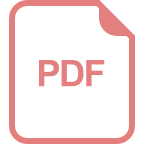
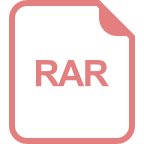
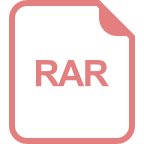
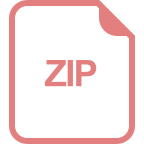
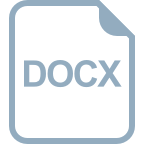
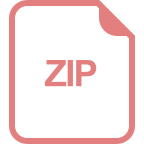
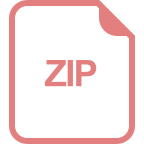
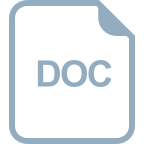
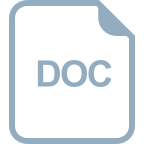
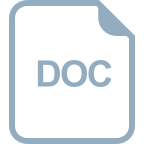





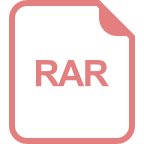