springBoot使用aop
时间: 2023-11-20 17:55:17 浏览: 121
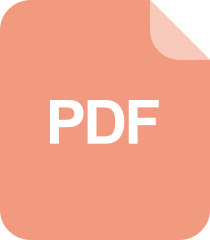
springboot的aop使用
在Spring Boot中使用AOP可以通过以下步骤实现:
1.在pom.xml文件中添加aop依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
```
2.创建一个切面类,使用@Aspect注解标注该类为切面类,并在该类中定义切点和通知:
```java
@Aspect
@Component
public class MyAspect {
@Pointcut("execution(* com.example.demo.service..*.*(..))")
public void pointcut() {}
@Before("pointcut()")
public void before(JoinPoint joinPoint) {
// 在方法执行前执行的逻辑
}
@After("pointcut()")
public void after(JoinPoint joinPoint) {
// 在方法执行后执行的逻辑
}
}
```
3.在切面类中定义切点和通知,切点用于定义哪些方法需要被拦截,通知用于定义拦截后执行的逻辑。
4.在需要使用AOP的类或方法上添加注解,例如@Log注解:
```java
@Service
public class UserService {
@Log
public void addUser(User user) {
// 添加用户的逻辑
}
}
```
5.在启动类上添加@EnableAspectJAutoProxy注解开启AOP功能:
```java
@SpringBootApplication
@EnableAspectJAutoProxy
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
阅读全文
相关推荐



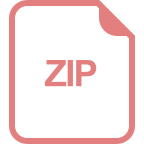
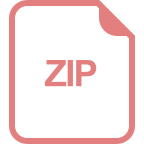
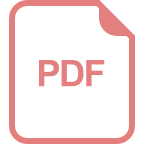
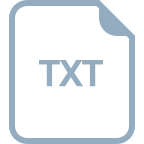
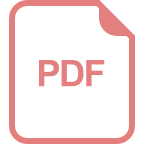







