npm如何安装d3.js
时间: 2024-08-12 08:03:09 浏览: 55
要在Node.js环境中使用D3.js,你需要全局安装它,因为D3.js主要用于浏览器环境。以下是安装D3.js的步骤:
1. 打开终端或命令提示符(取决于你的操作系统)。
2. 确保已经安装了`npm`(Node包管理器)。如果没有,可以访问 https://nodejs.org 下载并按照指示安装。
3. 在命令行输入以下命令进行全局安装:
```bash
npm install -g d3
```
这里的 `-g` 标志表示全局安装。如果安装成功,`d3` 就会在你的系统路径中可用,不需要在项目文件夹里引入。
如果你想要在某个特定的项目中使用D3.js,只需要在项目的`package.json`文件中添加对D3.js的依赖:
```json
{
"dependencies": {
"d3": "^7.0.0" // 最新版本或其他指定版本
}
}
```
然后运行 `npm install` 来安装该项目内的D3.js依赖。
完成上述步骤后,你就可以在项目的JavaScript文件中导入并使用D3.js了。
相关问题
uniapp npm 安装d3.js
在uniapp中使用npm安装d3.js,可以按照以下步骤进行操作:
1. 打开你的uniapp项目,在项目根目录下找到`package.json`文件,确保你的项目已经初始化为npm项目。
2. 打开终端或命令行工具,进入到你的uniapp项目根目录。
3. 运行以下命令来安装d3.js:
```
npm install d3
```
这将会在你的项目中安装d3.js及其相关依赖。
4. 安装完成后,在你的uniapp项目中,你可以通过以下方式引入d3.js:
```javascript
import * as d3 from 'd3';
```
这样就可以在你的uniapp项目中使用d3.js的功能了。
需要注意的是,uniapp是基于Vue的跨平台框架,而d3.js是一个用于数据可视化的JavaScript库,它并不是专门为uniapp设计的。因此,在使用d3.js时,可能需要根据uniapp和Vue的特性进行一些适配和调整。
D3.js 怎样npm
1. 安装Node.js:首先需要在官网下载并安装Node.js,安装完成后可以在命令行中输入node -v来检查是否安装成功。
2. 安装npm:npm是Node.js的包管理工具,可以通过命令行输入npm -v来检查是否已经安装。如果没有安装,可以通过命令行输入npm install npm -g来进行安装。
3. 配置npm镜像:由于npm默认的镜像源在国外,下载速度较慢,可以通过命令行输入npm config set registry https://registry.npm.taobao.org来将镜像源切换为淘宝镜像源。
4. 安装全局模块:可以通过命令行输入npm install -g 模块名来安装全局模块,例如npm install -g express。
5. 创建项目:可以通过命令行输入npm init来创建一个新的项目,根据提示输入相关信息即可。
6. 安装项目依赖:在项目目录下,可以通过命令行输入npm install 模块名来安装项目依赖,例如npm install express。
7. 配置package.json:在项目目录下,可以通过编辑package.json文件来配置项目信息和依赖。
8. 运行项目:在项目目录下,可以通过命令行输入node app.js来运行项目。
相关推荐
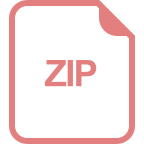
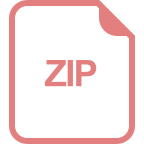
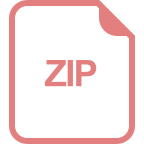
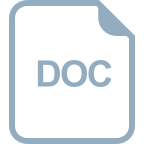
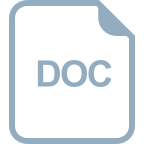
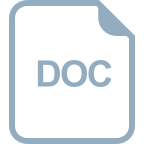
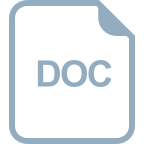
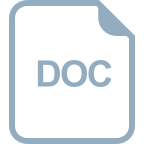
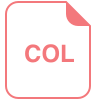
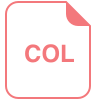
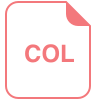
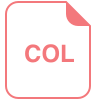
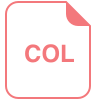


