我有一个qt项目,有一个pcap格式的离线文件叫123.pcap, 用C++的二进制方式解析它,使用fopen打开它,用fread读取它, 然后解析出每一个数据包中的协议号,检查所有的UDP类型协议及其他UDP类型的协议的数据包,其余协议的数据包迭丢弃不要。 然后再从每个udp数据包中的解析出,发送时间,目标ip,目标端口。 并然后打印,协议号,发送时间,目标ip,目标端口。 然后用 QUdpSocket::writeDatagram 方法将过滤出来的udp数据包发送给解析出来的目标ip,目标端口。 在函数 parsePcapFile_1 中实现上述的功能。 要求不能使用pcap的第三方库。 要求不要自己定义结构体。
时间: 2024-04-13 13:31:07 浏览: 148
您可以按照以下步骤在函数 `parsePcapFile_1` 中实现对 pcap 文件的解析和过滤操作:
1. 使用 `fopen` 打开 pcap 文件("123.pcap")并检查是否成功打开。
2. 使用 `fread` 读取 pcap 文件头部,并检查是否成功读取。
3. 循环读取每个数据包,直到文件结束:
- 使用 `fread` 读取数据包头部,并检查是否成功读取。
- 解析数据包中的协议号,您可以根据 pcap 文件格式(例如,以太网头部、IP头部等)来获取协议号。
- 如果协议号为 UDP 类型,则继续解析数据包。
- 从 UDP 数据包中解析发送时间、目标 IP 和目标端口。
- 打印协议号、发送时间、目标 IP 和目标端口。
- 使用 `QUdpSocket::writeDatagram` 方法将过滤出来的 UDP 数据包发送给目标 IP 和目标端口。
4. 关闭 pcap 文件。
下面是一个示例代码,可以帮助您开始实现 `parsePcapFile_1` 函数:
```cpp
#include <stdio.h>
#include <stdint.h>
void parsePcapFile_1()
{
const char* filename = "123.pcap";
FILE* file = fopen(filename, "rb");
if (!file) {
printf("Failed to open file: %s\n", filename);
return;
}
// Read pcap file header
uint8_t pcapHeader[24];
size_t bytesRead = fread(pcapHeader, 1, sizeof(pcapHeader), file);
if (bytesRead != sizeof(pcapHeader)) {
printf("Failed to read pcap file header\n");
fclose(file);
return;
}
// Loop through each packet
while (!feof(file)) {
// Read packet header
uint8_t packetHeader[16];
bytesRead = fread(packetHeader, 1, sizeof(packetHeader), file);
if (bytesRead != sizeof(packetHeader)) {
break;
}
// Parse protocol number
// Your code to extract protocol number goes here
// Check if it's a UDP packet
// Your code to check if the protocol number is UDP goes here
// Read UDP payload
uint8_t udpPayload[/* your expected payload size */];
bytesRead = fread(udpPayload, 1, sizeof(udpPayload), file);
if (bytesRead != sizeof(udpPayload)) {
break;
}
// Parse sending time, destination IP and destination port
// Your code to parse sending time, destination IP and destination port goes here
// Print protocol number, sending time, destination IP and destination port
// Your code to print the parsed information goes here
// Send UDP packet using QUdpSocket::writeDatagram
// Your code to send the UDP packet goes here
}
fclose(file);
}
```
请注意,此示例代码仅提供了一个框架,您需要根据具体的 pcap 文件格式和数据结构进行适当的解析和处理。
阅读全文
相关推荐
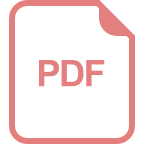

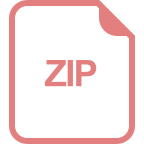
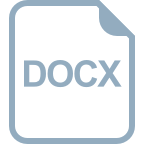
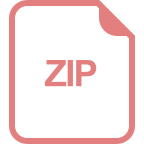
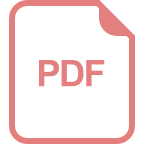
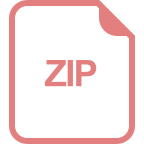
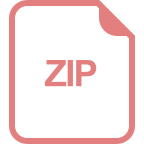
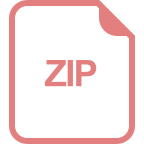
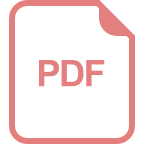
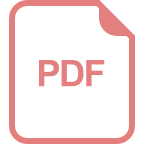



