如何设计一个程序,在父进程中输出每个子进程(线程)的学号和进程ID(线程名称),同时子进程中输出对应的姓名拼音以及其自身的进程ID(线程名称)?
时间: 2024-10-21 17:17:38 浏览: 40
为了设计这样一个程序,你可以考虑使用Unix/Linux系统调用,如`fork()`、`exec()`、`wait()`和`printf()`等,配合C语言或者Python等支持多线程的语言。这里给出一个简单的Python示例(因为Python有内置的多线程模块和易于理解的语法):
```python
import os
import pty
import subprocess
from concurrent.futures import ThreadPoolExecutor
# 父进程函数
def parent_process(child_pid):
# 获取子进程的状态
child_status = os.waitpid(child_pid, 0)
print(f"子进程{child_pid} (学号) 结束,退出状态码 {child_status[1]}")
# 输出子进程信息
with open(f"/proc/{child_pid}/status", "r") as proc_file:
for line in proc_file:
if "Threads:" in line:
threads_info = line.split()[1]
print(f"子进程{child_pid}: 子线程数量 {threads_info}")
# 子进程函数
def child_process(name):
# 拼音转换库(假设有一个名为pinyin的模块)
from pypinyin import lazy_pinyin
print(f"子进程启动,PID: {os.getpid()},姓名:{name}")
# 将姓名转换成拼音并输出
pinyin_name = ' '.join(lazy_pinyin(name))
print(f"子进程{os.getpid()},姓名拼音:{pinyin_name}")
if __name__ == "__main__":
# 创建子进程池
executor = ThreadPoolExecutor(max_workers=2)
# 使用pty模块模拟终端环境,便于输出
master_fd, slave_fd = pty.openpty()
# 分别创建两个子进程,并将它们的输出重定向到父进程的管道
child1 = subprocess.Popen(["python", "-c", f"child_process('张三')"], stdout=slave_fd, stderr=slave_fd)
child2 = subprocess.Popen(["python", "-c", f"child_process('李四')"], stdout=slave_fd, stderr=slave_fd)
# 添加任务到子进程池
executor.submit(parent_process, child1.pid)
executor.submit(parent_process, child2.pid)
# 关闭子进程的管道
os.close(slave_fd)
# 主进程等待所有子进程结束
executor.shutdown()
```
这个示例展示了如何在一个环境中让父进程监控子进程及其线程信息,同时子进程输出自己的名字拼音。注意实际项目中可能需要处理异常情况,并且在Windows上可能会有不同的系统调用。
阅读全文
相关推荐
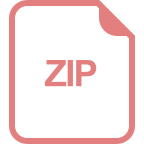
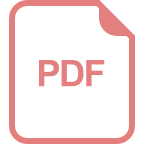
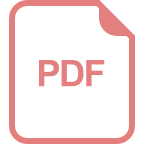
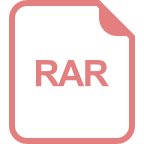
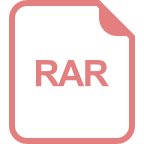
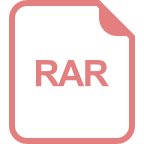
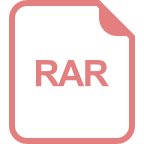
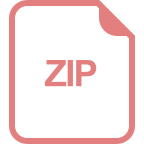
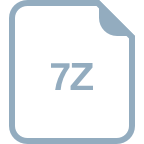
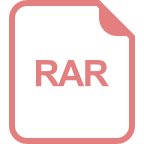
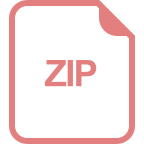
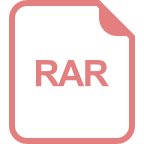
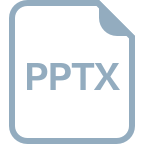
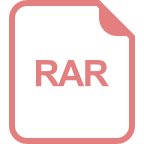
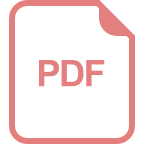
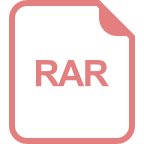
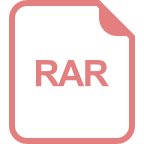
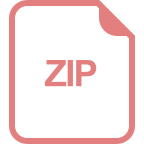