C语言,如何将读取的一串数据分别读取出来,并发送出来,举例子详细注解
时间: 2024-12-02 21:22:06 浏览: 5
在C语言中,如果从某个串口设备读取到一串二进制数据,通常会先将其存储在一个数组或其他类型的结构体中,然后再逐个处理这些数据元素。这里假设我们已经有一个包含数据的数组,接下来的例子将演示如何读取并逐个发送这些数据。
```c
#include <stdio.h>
#include <unistd.h> // 包含read和write函数
#include <fcntl.h> // 包含文件描述符相关操作
#include <termios.h> // 对于串口配置
// 假设我们有一组数据存储在char数组data[]中
const char *data = "This is an example of binary data";
int num_bytes = strlen(data); // 数据长度
// 串口配置和打开
#define SERIAL_PORT "/dev/ttyS0"
int fd;
struct termios options;
if ((fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY)) == -1) {
perror("Failed to open serial port");
exit(1);
}
// 设置串口属性
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率,比如9600 bps
cfsetospeed(&options, B9600);
cfmakeraw(&options);
tcflush(fd, TCIFLUSH); // 清空输入缓冲区
tcsetattr(fd, TCSANOW, &options);
// 遍历数据并逐字节发送
for (int i = 0; i < num_bytes; i++) {
int byte_to_send = data[i]; // 提取当前字节
write(fd, &byte_to_send, 1); // 写入串口
usleep(10000); // 短暂延时,模拟发送间隔
printf("Sent byte %d: %x\n", i, byte_to_send); // 输出发送过程
}
// 关闭串口
close(fd);
printf("Finished sending all data.\n");
阅读全文
相关推荐



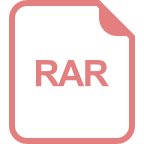
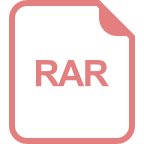
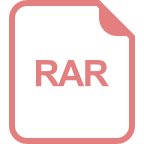
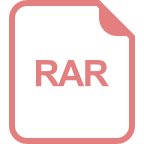
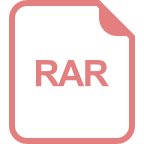
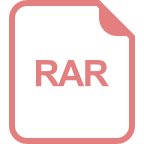

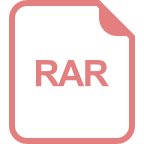
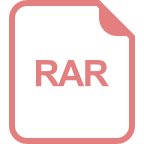







