数据结构作业: 寻找顺序表,链表应用的实际场景并编码实现(用c语言)
时间: 2024-10-20 22:11:08 浏览: 27
顺序表和链表都是数据结构的基本概念,在实际编程中有着广泛的应用。下面简单解释一下它们及其应用场景,并给出基本的C语言实现。
**顺序表**(如数组):
- **应用场景**:常用于存储固定大小的数据集合,如静态内存分配、快速随机访问元素(通过索引)等。
- **编码实现** (假设为整型数组):
```c
#include <stdio.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int size;
} SequenceList;
// 插入元素到序列表的末尾
void insert(SequenceList* list, int value) {
if (list->size == MAX_SIZE) {
printf("列表已满,无法插入。\n");
return;
}
list->data[list->size++] = value;
}
// 查找元素
int search(SequenceList* list, int target) {
for (int i = 0; i < list->size; i++) {
if (list->data[i] == target) {
return i;
}
}
return -1; // 如果未找到,返回-1
}
// 示例
int main() {
SequenceList my_list;
// 初始化...
insert(&my_list, 5);
int index = search(&my_list, 5); // 查找5的位置
return 0;
}
```
**链表**(如单向链表):
- **应用场景**:动态分配内存,适合频繁插入/删除元素的操作,因为无需移动大量元素;比如文件系统中的目录结构、浏览器历史记录等。
- **编码实现** (单链表节点和部分函数示例):
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node* next;
} LinkNode;
// 创建新节点
LinkNode* create_node(int value) {
LinkNode* newNode = malloc(sizeof(LinkNode));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 插入节点到链表
void insert_link(List* list, int value) {
LinkNode* newNode = create_node(value);
if (!list->head) {
list->head = newNode;
} else {
LinkNode* current = list->head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// 遍历链表查找
LinkNode* find_in_list(List* list, int target) {
LinkNode* current = list->head;
while (current) {
if (current->data == target) {
return current;
}
current = current->next;
}
return NULL;
}
// 示例
struct List {
LinkNode* head;
};
int main() {
List myList;
// 初始化...
insert_link(&myList, 5);
LinkNode* foundNode = find_in_list(&myList, 5); // 查找5的节点
return 0;
}
```
阅读全文
相关推荐
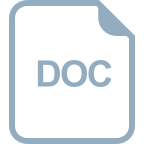
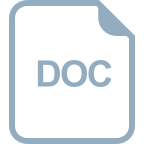
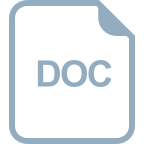

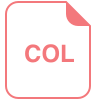
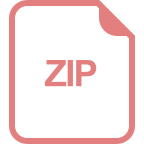
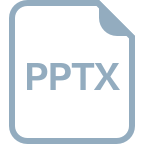
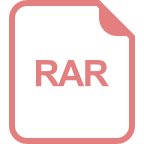
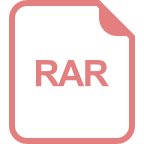
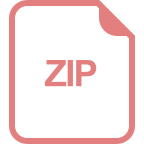
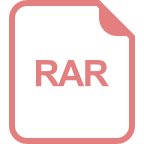
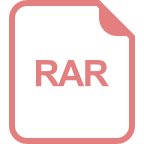
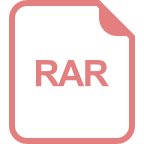
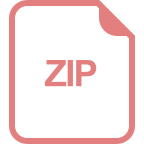
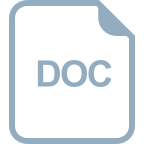
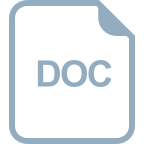
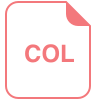
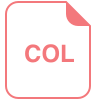
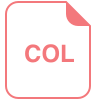