用python写代码,对给出的文本进行特征提取与分析,对数据进行预处理,并保存到新文件中。
时间: 2024-09-22 08:08:26 浏览: 67
在Python中,我们可以使用各种库来进行文本特征提取和分析,比如`nltk`(自然语言工具包)、`scikit-learn`、`spaCy`等。以下是一个基本示例,展示如何对文本进行预处理和特征提取,然后将其保存到新的CSV文件中:
首先确保已经安装了这些库,如果没有,可以通过`pip install nltk scikit-lemmatizer spacy`命令安装。
```python
import pandas as pd
import nltk
from nltk.corpus import stopwords
from sklearn.feature_extraction.text import CountVectorizer, TfidfVectorizer
from sklearn.pipeline import Pipeline
import string
# 下载必要的nltk资源(如果还没有下载)
nltk.download('punkt')
nltk.download('stopwords')
# 定义预处理步骤
def preprocess_text(text):
# 将文本转换为小写
text = text.lower()
# 移除标点符号
text = text.translate(str.maketrans('', '', string.punctuation))
# 分词
tokens = nltk.word_tokenize(text)
# 删除停用词
stop_words = set(stopwords.words('english'))
tokens = [token for token in tokens if token not in stop_words]
# 词干提取(这里使用简单的Lemmatization)
lemmatizer = nltk.WordNetLemmatizer()
tokens = [lemmatizer.lemmatize(token) for token in tokens]
return ' '.join(tokens)
# 假设我们有一个包含文本的数据框df
data = {
'text': ['这是第一个例子', '这是第二个句子', '第三个文本']
}
df = pd.DataFrame(data)
# 创建一个管道,将预处理和特征提取结合
vectorizer = TfidfVectorizer() # 或者CountVectorizer(),看需求
pipeline = Pipeline([
('preprocessing', preprocessing),
('vectorizing', vectorizer)
])
# 对文本进行处理并保存结果
processed_data = pipeline.fit_transform(df['text'])
feature_names = vectorizer.get_feature_names_out()
# 将处理后的特征数据和列名放入一个新的DataFrame
new_df = pd.DataFrame(processed_data.toarray(), columns=feature_names)
# 保存到CSV文件
new_df.to_csv('preprocessed_data.csv', index=False)
```
阅读全文
相关推荐
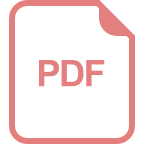
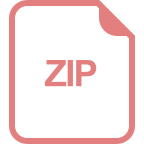
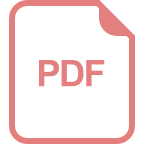















