stm32f103的usart1串口通信
时间: 2023-09-28 12:05:07 浏览: 60
你可以使用STM32F103微控制器的USART1串口进行通信。USART1是一个通用的异步同步收发器,可用于串行通信。首先,你需要配置串口的参数,如波特率、数据位数、停止位等。然后,你可以使用相应的函数来发送和接收数据。
以下是一个简单的示例代码,演示了如何配置和使用USART1进行串口通信:
```c
#include "stm32f10x.h"
void USART1_Init(void)
{
// 使能串口1和GPIOA的时钟
RCC->APB2ENR |= RCC_APB2ENR_USART1EN | RCC_APB2ENR_IOPAEN;
// 配置USART1引脚
GPIOA->CRH |= GPIO_CRH_MODE9_1 | GPIO_CRH_CNF9_1; // TX引脚
GPIOA->CRH |= GPIO_CRH_CNF10_0; // RX引脚
// 配置USART1参数
USART1->BRR = 0x271; // 波特率为115200 (APB2时钟为72MHz)
USART1->CR1 = USART_CR1_TE // 使能发送
| USART_CR1_RE // 使能接收
| USART_CR1_UE; // 使能USART
// 清除标志位
USART1->SR = 0;
}
void USART1_SendChar(char ch)
{
while (!(USART1->SR & USART_SR_TXE)); // 等待发送缓冲区为空
USART1->DR = (ch & 0xFF); // 发送数据
}
char USART1_ReceiveChar(void)
{
while (!(USART1->SR & USART_SR_RXNE)); // 等待接收缓冲区非空
return (USART1->DR & 0xFF); // 读取接收到的数据
}
int main(void)
{
USART1_Init();
while (1)
{
char receivedChar = USART1_ReceiveChar();
// 处理接收到的数据
USART1_SendChar('A');
// 发送数据
}
}
```
你可以根据自己的需求修改代码,并根据需要添加错误检测和处理。希望这可以帮助到你!
相关推荐
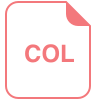
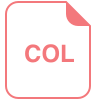
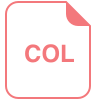
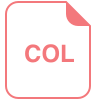
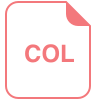









