c语言凯撒密码加解密程序代码
时间: 2024-10-28 22:03:59 浏览: 122
凯撒密码是一种简单的替换加密技术,通过将字母表中的每个字符按照固定位移进行替换。以下是使用C语言编写的一个基础的凯撒密码加解密程序代码示例:
```c
#include <stdio.h>
#include <ctype.h>
// 加密函数
char encrypt(char ch, int shift) {
if (isupper(ch)) { // 如果是大写字母
return ((ch - 'A' + shift) % 26) + 'A';
} else if (islower(ch)) { // 如果是小写字母
return ((ch - 'a' + shift) % 26) + 'a';
} else { // 非字母字符不变
return ch;
}
}
// 解密函数
char decrypt(char ch, int shift) {
return encrypt(ch, -shift); // 加密与解密位移相反
}
int main() {
char text[100], encrypted[100];
int shift, i;
printf("请输入明文:");
fgets(text, sizeof(text), stdin);
text[strlen(text)-1] = '\0'; // 去除换行符
printf("请输入位移(1-25):");
scanf("%d", &shift);
for (i = 0; text[i]; i++) {
encrypted[i] = encrypt(text[i], shift);
}
encrypted[strlen(encrypted)] = '\0'; // 添加结束符
printf("加密后的密文是:%s\n", encrypted);
printf("输入密文解密:");
gets(encrypted); // 注意:gets有安全风险,实际项目应使用fgets代替
for (i = 0; encrypted[i]; i++) {
text[i] = decrypt(encrypted[i], shift);
}
text[strlen(text)] = '\0';
printf("解密后的明文是:%s\n", text);
return 0;
}
```
在这个例子中,用户首先输入一段明文,然后选择一个位移值,程序会将明文按选定的位移进行加密并显示结果。接着用户可以输入密文进行解密。
阅读全文
相关推荐
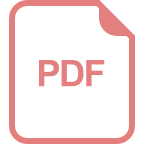
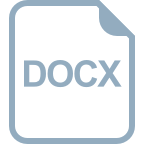
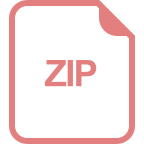















