pandas提取某一列显示 only integers, slices (`:`), ellipsis (`...`), numpy.newaxis (`None`) and integer or boolean arrays are valid indices
时间: 2023-11-21 21:50:08 浏览: 99
要提取某一列并且只显示整数值,您可以使用 `loc` 或 `iloc` 函数来完成。下面是两种方法的示例:
使用 `loc` 函数:
```python
import pandas as pd
# 创建一个示例数据框
data = {'A': [1, 2, 3, 4, 5],
'B': [1.1, 2.2, 3.3, 4.4, 5.5],
'C': [1, 2, 3, 4, 5]}
df = pd.DataFrame(data)
# 使用 loc 函数提取整数列
int_column = df.loc[:, df.dtypes == 'int64']
print(int_column)
```
使用 `iloc` 函数:
```python
import pandas as pd
# 创建一个示例数据框
data = {'A': [1, 2, 3, 4, 5],
'B': [1.1, 2.2, 3.3, 4.4, 5.5],
'C': [1, 2, 3, 4, 5]}
df = pd.DataFrame(data)
# 使用 iloc 函数提取整数列
int_column = df.iloc[:, df.dtypes == 'int64']
print(int_column)
```
这两种方法都会提取数据框中所有整数类型的列,并且只显示整数值。
相关问题
IndexError: only integers, slices (`:`), ellipsis (`...`), numpy.newaxis (`None`) and integer or boolean arrays are valid indices
这个错误通常在使用Python中的索引时出现,原因是使用了无效的索引类型。
建议检查代码中的索引类型是否正确,例如使用了浮点数或字符串进行索引。如果需要使用非整数索引时,可以考虑使用Pandas库中的标签索引(Label-based indexing)或iloc方法。
另外,还有可能是在使用NumPy数组时出现了该错误,原因也是类似的。建议检查代码中对数组的索引是否正确,例如是否使用了非整数类型或者超出了数组的索引范围等问题。
test1 = pd.read_csv('./test_cleaned1.csv') # 导入测试数据集 features=test1.keys() test = pd.read_csv('./happiness_test_abbr.csv') test.set_index(test.columns[0], inplace=True) # 设置索引列 test=test[list(features[1:])].values # test=test.iloc[0:,:] y_predict = model.predict(test) test['happiness'] = y_predict submit = test[['id', 'happiness']] print(submit)only integers, slices (:), ellipsis (...), numpy.newaxis (None) and integer or boolean arrays are valid indices
这个错误提示通常是在使用 Pandas DataFrame 对象进行索引时,使用了不合法的类型。合法的类型包括整数、切片(`:`)、省略号(`...`)、`numpy.newaxis`、整数或布尔类型的数组。请检查你的代码是否在对 DataFrame 进行索引时,使用了不合法的类型。另外,如果你需要更多帮助,请提供更具体的代码和错误信息。
阅读全文
相关推荐
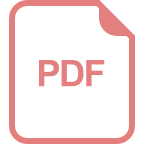
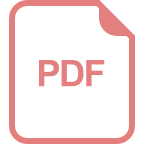
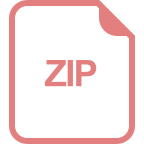
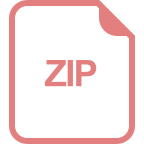
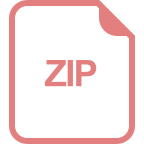
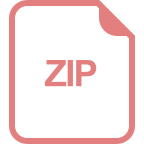
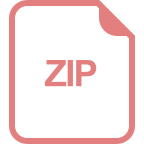
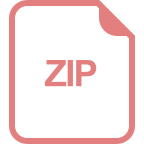
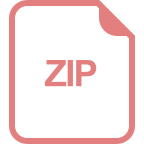
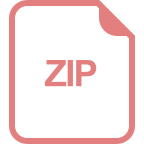
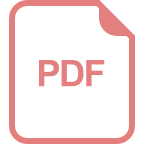
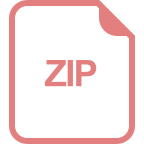
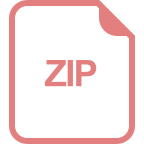
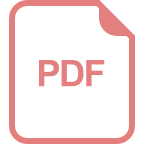
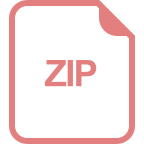
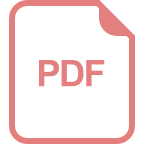