请你给我设计的完整代码
时间: 2024-11-19 14:36:10 浏览: 17
在设计一个完整的聊天机器人的代码时,我们通常会使用Python,并结合像TensorFlow或PyTorch这样的深度学习库。以下是一个基础的示例,展示了如何使用Keras库构建一个简单的seq2seq聊天机器人模型:
```python
# 导入库
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, LSTM, Dense, RepeatVector, TimeDistributed
# 定义数据预处理函数
def preprocess_data(data):
# ... (这里处理文本数据,例如分词、编码)
return encoded_data, tokenizer
# 负责编码和解码的函数
def encode_decode(model, input_seq, output_seq, max_len):
encoder_inputs = input_seq
decoder_inputs = tf.expand_dims([tokenizer.word_index['<start>']] + [word for word in output_seq], axis=0)
# 解码器的初始隐藏状态等于编码器的最后一层隐藏状态
hidden_state = [tf.zeros((1, units)), tf.zeros((1, units))]
decoded_sentence = ''
for t in range(1, max_len):
predictions, hidden_state = model(encoder_inputs, hidden_state)
sampled_word_index = tf.argmax(predictions[0, t-1, :], axis=-1)
sampled_word = inverse_tokenizer.index_word(sampled_word_index.numpy())
if sampled_word == '<end>':
break
decoded_sentence += ' ' + sampled_word
decoder_inputs = tf.concat([decoder_inputs, [[sampled_word_index]]], axis=-1)
return decoded_sentence
# 参数设置
input_length, units = 100, 256
max_decoder_sequence_length = 50
# 构建模型
encoder_input = Input(shape=(None,))
encoder = LSTM(units, return_state=True)
encoder_outputs, state_h, state_c = encoder(encoder_input)
# 序列解码
decoder_input = RepeatVector(max_decoder_sequence_length)(state_h)
decoder_lstm = LSTM(units, return_sequences=True, return_state=True)
decoder_outputs, _, _ = decoder_lstm(decoder_input, initial_state=[state_h, state_c])
decoder_dense = Dense(len(tokenizer.word_index)+1, activation='softmax')
decoder_outputs = decoder_dense(decoder_outputs)
model = Model(inputs=encoder_input, outputs=decoder_outputs)
# 编译并训练模型
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy')
... (这里添加训练代码)
# 使用模型进行预测
input_text = "你好"
output_text = encode_decode(model, input_text_to_encode, [], max_len)
print(output_text)
```
这只是一个基础的模板,实际应用中还需要根据数据集的特性调整参数、实现数据加载、批次处理等功能,并可能需要更复杂的模型结构和技术来提升性能。记得在项目的每个阶段都要保存检查点以便于调试和继续训练。
阅读全文
相关推荐
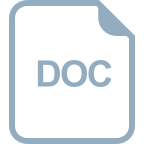
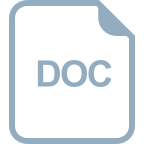
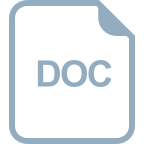
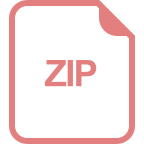
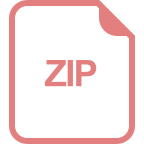
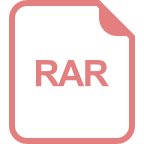
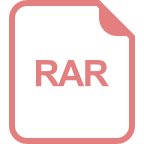
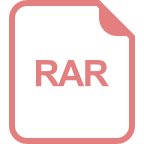
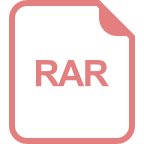
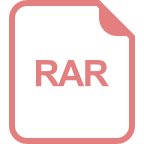
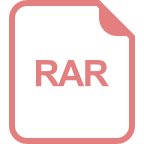
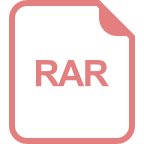
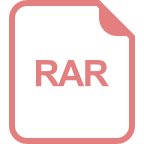
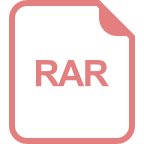
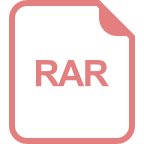