html给表格每个单元格绑定上下左右键盘事件,包含具体逻辑的js代码
时间: 2024-09-19 12:10:09 浏览: 47
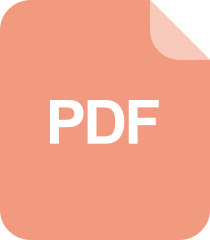
键盘上下键移动选择table表格行的js代码
HTML表格中的每个单元格通常不会直接绑定键盘事件,因为它们并不是DOM元素的原生行为。但是,你可以通过JavaScript来模拟这种功能。下面是一个基本的例子,展示了如何为表格的每个单元格添加键盘监听,支持上、下、左、右四个方向:
```html
<table id="myTable">
<tr>
<td>Cell 1</td>
<td>Cell 2</td>
</tr>
<!-- 更多行... -->
</table>
<script>
// 获取表格
const table = document.getElementById('myTable');
const cells = Array.from(table.getElementsByTagName('td'));
// 定义移动函数,这里只是一个简化示例,实际应用中需要考虑边界情况
function moveCell(direction) {
const selectedCell = document.activeElement;
if (selectedCell) {
let nextIndex = selectedCell.cellIndex + (direction === 'up' ? -1 : direction === 'down' ? 1 : direction === 'left' ? -1 : 1);
// 确保索引在范围内
if (nextIndex >= 0 && nextIndex < cells.length) {
selectedCell.blur();
cells[nextIndex].focus();
}
}
}
// 绑定键盘事件
cells.forEach(cell => {
cell.addEventListener('keydown', event => {
switch (event.key) {
case 'ArrowUp':
moveCell('up');
break;
case 'ArrowDown':
moveCell('down');
break;
case 'ArrowLeft':
moveCell('left');
break;
case 'ArrowRight':
moveCell('right');
break;
default:
break; // 如果不是指定的方向键,则不做处理
}
});
});
</script>
```
在这个例子中,我们首先获取了所有的`<td>`元素,然后为每个单元格添加了一个`keydown`事件监听器,根据箭头键的不同值触发相应的移动函数。
注意这仅是一个基础示例,实际场景中你可能需要更复杂的逻辑,比如处理表格外边界、嵌套表格等情况。
阅读全文
相关推荐
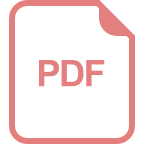
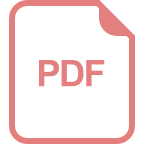



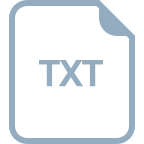
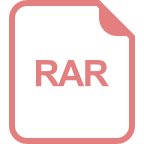
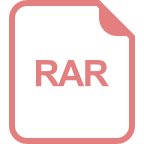
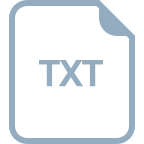
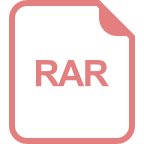
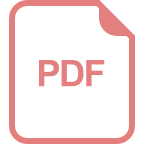
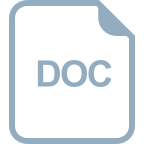
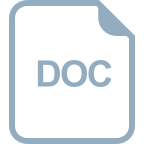
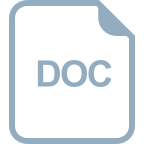
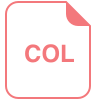
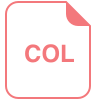

