三个线程交替打印abc
时间: 2023-09-02 22:05:58 浏览: 61
你可以使用多线程编程来实现三个线程交替打印abc。下面是一个示例代码:
```python
import threading
class PrintABC:
def __init__(self):
self.current_letter = 'A'
self.lock = threading.Lock()
def print_a(self):
for _ in range(10):
with self.lock:
while self.current_letter != 'A':
self.lock.wait()
print('A', end='')
self.current_letter = 'B'
self.lock.notify_all()
def print_b(self):
for _ in range(10):
with self.lock:
while self.current_letter != 'B':
self.lock.wait()
print('B', end='')
self.current_letter = 'C'
self.lock.notify_all()
def print_c(self):
for _ in range(10):
with self.lock:
while self.current_letter != 'C':
self.lock.wait()
print('C', end='')
self.current_letter = 'A'
self.lock.notify_all()
def main():
printer = PrintABC()
thread_a = threading.Thread(target=printer.print_a)
thread_b = threading.Thread(target=printer.print_b)
thread_c = threading.Thread(target=printer.print_c)
thread_a.start()
thread_b.start()
thread_c.start()
thread_a.join()
thread_b.join()
thread_c.join()
if __name__ == '__main__':
main()
```
这个例子中,我们创建了一个 `PrintABC` 类,其中包含了三个方法 `print_a`、`print_b` 和 `print_c` 分别用于打印字母 'A'、'B' 和 'C'。在每个方法中,使用 `with self.lock` 来获取锁对象并进入临界区域。通过 `self.current_letter` 来确定当前应该打印的字母,并使用 `while self.current_letter != 'A'` 等待其他线程改变 `self.current_letter` 的值。当当前字母符合要求时,打印字母并切换到下一个字母,然后使用 `self.lock.notify_all()` 唤醒其他等待的线程。
在 `main` 方法中,我们创建了三个线程并分别启动它们,然后使用 `join` 方法等待所有线程执行完毕。运行这段代码时,你将会看到三个线程交替打印字母 'A'、'B' 和 'C',每个字母连续打印十次。
相关推荐
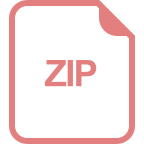
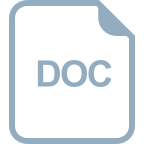
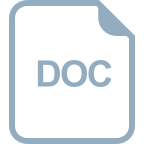














