python 射击游戏
时间: 2023-08-23 07:16:47 浏览: 89
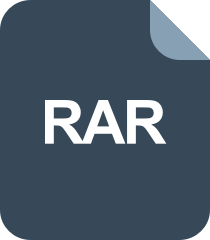
shoot-the-fruit1_pythonshootfruit_Python游戏_Shoot!_shoottheapple_
对于创建一个简单的Python射击游戏,你可以使用Pygame库。下面是一个基本的示例代码:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 设置游戏窗口大小
width = 800
height = 600
window = pygame.display.set_mode((width, height))
pygame.display.set_caption("射击游戏")
# 定义颜色
white = (255, 255, 255)
red = (255, 0, 0)
# 玩家的初始位置和速度
player_x = width // 2
player_y = height - 50
player_speed = 5
# 子弹的初始位置和速度
bullet_x = player_x
bullet_y = player_y
bullet_speed = 10
bullet_state = "ready"
# 敌人的初始位置和速度
enemy_x = random.randint(0, width)
enemy_y = random.randint(50, 150)
enemy_speed = 3
# 计分
score = 0
# 游戏主循环
running = True
while running:
# 填充背景颜色
window.fill(white)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 监听键盘按键事件
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player_x -= player_speed
if event.key == pygame.K_RIGHT:
player_x += player_speed
if event.key == pygame.K_SPACE:
if bullet_state == "ready":
bullet_x = player_x
bullet_y = player_y
bullet_state = "fire"
# 更新玩家位置
player_x = max(0, min(player_x, width - 64)) # 限制玩家在游戏窗口内移动
pygame.draw.rect(window, red, (player_x, player_y, 64, 64))
# 更新子弹位置
if bullet_state == "fire":
bullet_y -= bullet_speed
if bullet_y < 0:
bullet_state = "ready"
if bullet_state == "ready":
bullet_x = player_x
bullet_y = player_y
pygame.draw.rect(window, red, (bullet_x + 30, bullet_y - 10, 4, 10))
# 更新敌人位置
enemy_x += enemy_speed
if enemy_x > width or enemy_x < 0:
enemy_x = random.randint(0, width)
enemy_y = random.randint(50, 150)
pygame.draw.rect(window, red, (enemy_x, enemy_y, 64, 64))
# 检测碰撞
if bullet_state == "fire":
if bullet_x > enemy_x and bullet_x < enemy_x + 64 and bullet_y > enemy_y and bullet_y < enemy_y + 64:
score += 1
bullet_state = "ready"
enemy_x = random.randint(0, width)
enemy_y = random.randint(50, 150)
# 更新分数显示
font = pygame.font.Font(None, 36)
text = font.render("Score: " + str(score), True, red)
window.blit(text, (10, 10))
# 更新屏幕显示
pygame.display.update()
# 退出游戏
pygame.quit()
```
这只是一个简单的示例,你可以根据自己的需求进行修改和扩展。希望对你有所帮助!
阅读全文
相关推荐
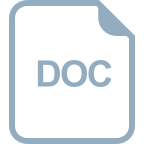
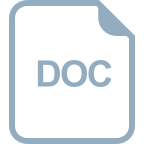

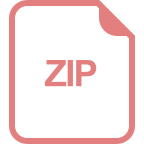



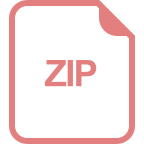
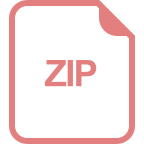
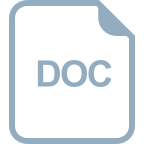
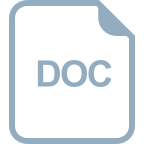





