std::cin >> n
时间: 2023-08-20 12:04:29 浏览: 54
您好!对于这个问题,我会假设您想要从用户输入中读取一个整数,并将其存储在变量n中。要实现这一点,您可以使用C++中的标准输入流std::cin和提取运算符>>。下面是一个示例代码:
```cpp
#include <iostream>
int main() {
int n;
std::cout << "请输入一个整数:";
std::cin >> n;
std::cout << "您输入的整数是:" << n << std::endl;
return 0;
}
```
在此示例中,我们首先向用户显示一条提示消息,要求用户输入一个整数。然后,使用std::cin和>>运算符将用户输入的整数存储在变量n中。最后,我们将该整数打印出来以进行确认。
请注意,您可以根据需要修改提示消息和打印输出的格式。希望这可以帮助到您!如果您有任何其他问题,请随时提问。
相关问题
找出下列代码的错误并加以解释 #include<iostream> using namespace std; template<typename T> int Search(T nValues[],int n,T d){ int i; for(i=0; i<n ;i++){ if(nValues[i] == d) { return i; }else{ return -1; } } } int main() { int n; std::cin >> n; int *nValues = new int[n]; for (int i = 0; i < n; i++) { std::cin >> nValues[i]; } int d; std::cin >> d; std::cout << Search(nValues, n, d) << std::endl; delete[] nValues; double f; std::cin >> n; double *dValues = new double[n]; for (int i = 0; i < n; i++) { std::cin >> dValues[i]; } std::cin >> f; std::cout << Search(dValues, n, f) << std::endl; delete[] dValues; std::cin >> n; char *cValues = new char[n]; for (int i = 0; i < n; i++) { std::cin >> cValues[i]; } char c; std::cin >> c; std::cout << Search(cValues, n, c) << std::endl; delete[] cValues; return 0; }
该代码存在以下错误:
1. 在模板函数Search中,如果找到指定元素,应该直接返回下标i,而不是返回i后再返回-1。因为这里的return -1会导致函数在找到第一个不匹配的元素后就立即返回-1,而不是继续查找下一个元素。
2. 在第三次调用Search函数时,应该使用char类型的模板参数,而不是默认的T。因为字符数组和其他类型的数组不同,字符数组需要按照字符的方式进行比较,而不是按照数值的方式进行比较。
修改后的代码如下:
```
#include <iostream>
using namespace std;
template<typename T>
int Search(T nValues[], int n, T d) {
for (int i = 0; i < n; i++) {
if (nValues[i] == d) {
return i;
}
}
return -1;
}
template<>
int Search(char nValues[], int n, char d) {
for (int i = 0; i < n; i++) {
if (nValues[i] == d) {
return i;
}
}
return -1;
}
int main() {
int n;
std::cin >> n;
int *nValues = new int[n];
for (int i = 0; i < n; i++) {
std::cin >> nValues[i];
}
int d;
std::cin >> d;
std::cout << Search(nValues, n, d) << std::endl;
delete[] nValues;
double f;
std::cin >> n;
double *dValues = new double[n];
for (int i = 0; i < n; i++) {
std::cin >> dValues[i];
}
std::cin >> f;
std::cout << Search(dValues, n, f) << std::endl;
delete[] dValues;
std::cin >> n;
char *cValues = new char[n];
for (int i = 0; i < n; i++) {
std::cin >> cValues[i];
}
char c;
std::cin >> c;
std::cout << Search(cValues, n, c) << std::endl;
delete[] cValues;
return 0;
}
```
修改后的代码中,我们使用了特化模板来处理字符数组的查找,这样就可以按照字符的方式进行比较,并且返回正确的下标值。
写出3组Plus函数的声明与实现,类型分别为int,double,string。 主函数如下,注意只需要实现Plus函数和包含相关的头文件。 int main() { int n; int a,b; double c,d; std::string str1,str2; std::cin>>n; while(n--) { std::cin >> a >> b ; std::cin >> c >> d; std::cin >> str1 >> str2; std::cout << myplus(a, b) << endl; std::cout << myplus(d, c) << endl; std::cout << myplus(str1, str2) << endl; } return 0; } Sample Input 1 2 3 2.5 3.5 women day Sample Output 5 6 womenday Hint PS:Please add std:: before using the string type.
int类型的声明与实现:
声明:
```
int myplus(int a, int b);
```
实现:
```
int myplus(int a, int b)
{
return a + b;
}
```
double类型的声明与实现:
声明:
```
double myplus(double a, double b);
```
实现:
```
double myplus(double a, double b)
{
return a + b;
}
```
string类型的声明与实现:
声明:
```
std::string myplus(std::string str1, std::string str2);
```
实现:
```
std::string myplus(std::string str1, std::string str2)
{
return str1 + str2;
}
```
完整代码如下:
```
#include <iostream>
#include <string>
int myplus(int a, int b)
{
return a + b;
}
double myplus(double a, double b)
{
return a + b;
}
std::string myplus(std::string str1, std::string str2)
{
return str1 + str2;
}
int main()
{
int n;
int a, b;
double c, d;
std::string str1, str2;
std::cin >> n;
while (n--)
{
std::cin >> a >> b;
std::cin >> c >> d;
std::cin >> str1 >> str2;
std::cout << myplus(a, b) << std::endl;
std::cout << myplus(d, c) << std::endl;
std::cout << myplus(str1, str2) << std::endl;
}
return 0;
}
```
相关推荐
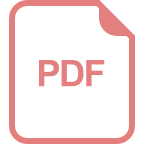
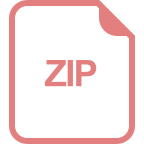
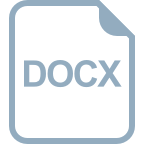












