如何使用C语言读取并解析256级灰度BMP图片的文件头和位图信息头,以及如何处理图像数据对齐?
时间: 2024-11-05 15:20:16 浏览: 48
解析256级灰度BMP图片的文件头和位图信息头,以及处理图像数据对齐是图像处理和文件读取的重要步骤。首先,我们需要阅读和理解文件头`BITMAPFILEHEADER`,这包含了文件的类型标识、文件大小、以及数据块的偏移量。接着,通过位图信息头`BITMAPINFOHEADER`获取图像的尺寸、颜色深度等信息。对于256级灰度图,`biBitCount`为8,表示每个像素占用1字节。调色板是解析BMP图片时的另一个关键部分,它描述了灰度级对应的RGB值。在读取完头信息和调色板后,接下来是处理图像数据的对齐问题,以保证行数据的字节对齐。在C语言中,通常使用位运算来实现对齐,确保数据存储的连续性和效率。具体到代码实现,可以创建对应的结构体来定义文件头和信息头,通过文件I/O函数读取和解析这些结构,同时利用位运算实现数据对齐。深入理解并掌握这些细节,能够使你在处理图像文件时更加得心应手。关于更深入的学习和实践,可以参考《C语言详解256级灰度BMP图片解析与写入》,这本书详细介绍了如何使用C语言进行BMP图片的解析和写入,是一份宝贵的资源。
参考资源链接:[C语言详解256级灰度BMP图片解析与写入](https://wenku.csdn.net/doc/68av5chiyy?spm=1055.2569.3001.10343)
相关问题
c语言读取8bit灰度图像的bmp文件
以下是读取8位灰度图像的bmp文件的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#pragma pack(push, 1) // 禁用对齐
struct bmp_file_header {
uint16_t magic_num; // 文件类型标识符
uint32_t size; // 文件大小
uint16_t reserved1; // 保留字段1
uint16_t reserved2; // 保留字段2
uint32_t offset; // 数据偏移量
};
struct bmp_info_header {
uint32_t header_size; // 位图信息头大小
int32_t width; // 图像宽度
int32_t height; // 图像高度
uint16_t planes; // 颜色平面数
uint16_t bit_depth; // 颜色位深度
uint32_t compression; // 压缩类型
uint32_t image_size; // 压缩图像大小
int32_t x_resolution; // 水平分辨率
int32_t y_resolution; // 垂直分辨率
uint32_t color_palette; // 调色板颜色数
uint32_t important_colors; // 重要颜色数
};
#pragma pack(pop) // 恢复对齐
int main() {
FILE *fp = fopen("example.bmp", "rb");
if (!fp) {
perror("failed to open file");
exit(1);
}
// 读取文件头信息
struct bmp_file_header file_header;
fread(&file_header, sizeof(file_header), 1, fp);
// 读取位图信息头信息
struct bmp_info_header info_header;
fread(&info_header, sizeof(info_header), 1, fp);
// 确保是8位灰度图像
if (info_header.bit_depth != 8) {
fprintf(stderr, "Not an 8-bit grayscale image\n");
exit(1);
}
// 读取调色板信息
uint8_t palette[1024];
fread(palette, sizeof(palette), 1, fp);
// 读取像素数据
uint8_t *data = malloc(info_header.image_size);
fseek(fp, file_header.offset, SEEK_SET);
fread(data, info_header.image_size, 1, fp);
// 关闭文件
fclose(fp);
// 显示图像
// TODO: 使用第三方库或自行实现图像显示
// 释放内存
free(data);
return 0;
}
```
这段代码使用了C语言的文件读写API和结构体来解析bmp文件头、位图信息头和调色板信息,然后使用`malloc()`函数动态分配内存并读取像素数据。如果需要显示图像,可以使用第三方库或自行实现图像显示。
C语言存储灰度图像bmp文件‘’
以下是将灰度图像存储为bmp文件的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#pragma pack(push, 1) // 禁用对齐
struct bmp_file_header {
uint16_t magic_num; // 文件类型标识符
uint32_t size; // 文件大小
uint16_t reserved1; // 保留字段1
uint16_t reserved2; // 保留字段2
uint32_t offset; // 数据偏移量
};
struct bmp_info_header {
uint32_t header_size; // 位图信息头大小
int32_t width; // 图像宽度
int32_t height; // 图像高度
uint16_t planes; // 颜色平面数
uint16_t bit_depth; // 颜色位深度
uint32_t compression; // 压缩类型
uint32_t image_size; // 压缩图像大小
int32_t x_resolution; // 水平分辨率
int32_t y_resolution; // 垂直分辨率
uint32_t color_palette; // 调色板颜色数
uint32_t important_colors; // 重要颜色数
};
#pragma pack(pop) // 恢复对齐
int main() {
// 读取图像数据
// TODO: 替换成实际的图像数据
uint8_t *data = NULL;
int width = 640;
int height = 480;
// 打开文件,准备写入
FILE *fp = fopen("example.bmp", "wb");
if (!fp) {
perror("failed to open file");
exit(1);
}
// 计算文件大小和数据偏移量
uint32_t image_size = width * height;
uint32_t file_size = sizeof(struct bmp_file_header) + sizeof(struct bmp_info_header) + 1024 + image_size;
uint32_t offset = sizeof(struct bmp_file_header) + sizeof(struct bmp_info_header) + 1024;
// 写入文件头信息
struct bmp_file_header file_header = {
.magic_num = 0x4D42, // BM
.size = file_size,
.reserved1 = 0,
.reserved2 = 0,
.offset = offset
};
fwrite(&file_header, sizeof(file_header), 1, fp);
// 写入位图信息头信息
struct bmp_info_header info_header = {
.header_size = sizeof(struct bmp_info_header),
.width = width,
.height = height,
.planes = 1,
.bit_depth = 8,
.compression = 0,
.image_size = image_size,
.x_resolution = 0,
.y_resolution = 0,
.color_palette = 256,
.important_colors = 0
};
fwrite(&info_header, sizeof(info_header), 1, fp);
// 写入调色板信息
uint8_t palette[1024] = {0};
for (int i = 0; i < 256; i++) {
palette[i * 4] = i;
palette[i * 4 + 1] = i;
palette[i * 4 + 2] = i;
}
fwrite(palette, sizeof(palette), 1, fp);
// 写入像素数据
fwrite(data, image_size, 1, fp);
// 关闭文件
fclose(fp);
// 释放内存
free(data);
return 0;
}
```
这段代码使用了C语言的文件读写API和结构体来生成bmp文件头、位图信息头、调色板信息和像素数据,并将它们写入文件。其中,调色板信息使用了灰度调色板,即将256个颜色值(0-255)都设置为相同的灰度值,以便表示灰度图像。如果需要将其他类型的图像存储为bmp文件,可以相应地修改位图信息头和像素数据。
阅读全文
相关推荐
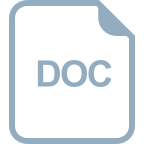
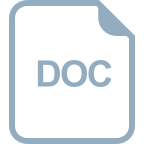
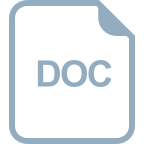
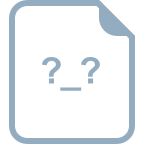
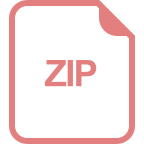
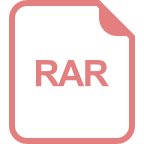
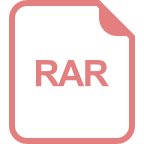
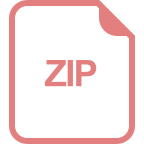
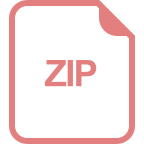
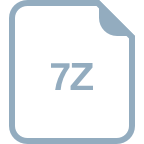
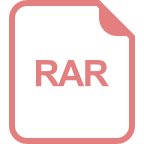
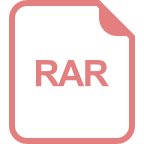
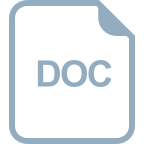



