使用Python完成下列项目决策任务 分析一个任务流程,给出最早完成时间和最晚完成时间,并可视化
时间: 2024-09-25 15:16:29 浏览: 31
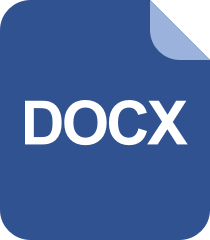
一个使用 Python 开发的简单数据可视化项目设计实例(含完整的程序和代码详解)
在Python中,我们可以利用一些库如Pandas、datetime和networkx或者图形化库如matplotlib和plotly来完成这个项目决策任务。首先,我们需要定义任务之间的依赖关系,比如通过一个字典或者DataFrame来表示每个任务及其开始和结束时间,以及它们之间的先后顺序。
假设我们有一个简单的任务列表和依赖关系数据结构:
```python
import pandas as pd
import networkx as nx
import datetime
# 示例任务数据
tasks = {
'A': {'start_time': datetime.datetime.now() - datetime.timedelta(hours=2), 'end_time': None},
'B': {'start_time': datetime.datetime.now(), 'end_time': None},
'C': {'start_time': datetime.datetime.now() + datetime.timedelta(hours=1), 'end_time': None},
'D': {'start_time': datetime.datetime.now() + datetime.timedelta(hours=3), 'end_time': None},
# 更复杂的任务添加在这里...
}
# 定义任务间的依赖关系
dependencies = [('A', 'B'), ('B', 'C'), ('C', 'D')]
# 创建有向图
G = nx.DiGraph()
for task, dep_data in tasks.items():
G.add_node(task)
if 'end_time' not in dep_data:
for dependency in dependencies:
if dependency[0] == task:
next_task = dependency[1]
G.add_edge(task, next_task)
# 找出最早完成时间和最晚完成时间
earliest_completion_time = min(G.nodes(data='start_time'))[1]['start_time']
latest_completion_time = max(nx.dag_longest_path_length(G) * [tasks[node]['end_time'] for node in nx.topological_sort(G)])
# 可视化任务流程
nx.draw_networkx(G, with_labels=True)
plt.title('Project Task Dependency Visualization')
plt.show()
print(f"最早完成时间: {earliest_completion_time}")
print(f"最晚完成时间: {latest_completion_time}")
```
在这个例子中,我们首先创建了一个有向图来表示任务间的依赖关系,然后找到最早和最晚完成时间,最后使用网络x库对任务流程进行可视化。
阅读全文
相关推荐
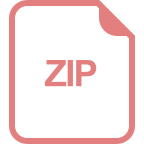
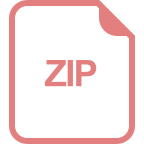
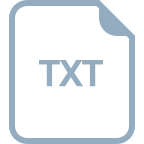
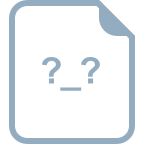
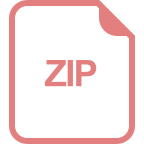
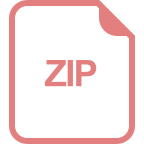
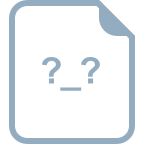
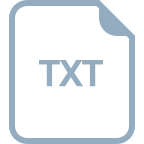
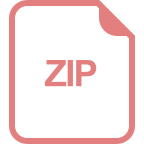
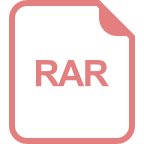
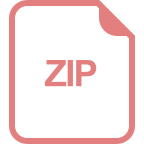
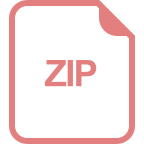
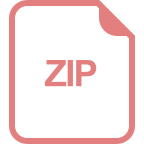
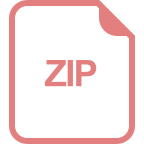
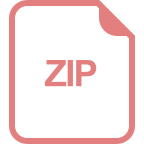
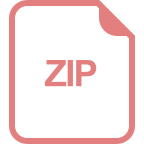
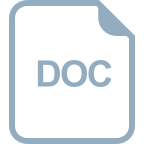
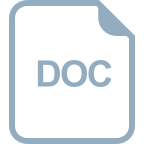