vue3watch监听多个参数
时间: 2024-05-24 07:07:52 浏览: 256
在Vue 3中,可以使用 `watch` 函数来监听多个参数的变化。具体实现方法如下:
1. 在 `setup` 函数中使用 `watch` 函数来监听多个参数的变化:
```javascript
import { watch } from 'vue';
export default {
setup() {
const state = reactive({
count: 0,
message: ''
});
// 监听count和message的变化
watch([() => state.count, () => state.message], ([newCount, newMessage], [oldCount, oldMessage]) => {
console.log(`count从${oldCount}变为${newCount},message从${oldMessage}变为${newMessage}`);
});
return { state };
}
}
```
2. 在 `watch` 函数中传入一个数组作为第一个参数,数组中包含了要监听的多个参数,第二个参数是一个回调函数,用来处理参数变化后的逻辑。
在回调函数中,第一个参数是新值的数组,第二个参数是旧值的数组,两者的顺序与第一个参数中监听的顺序一致。
相关问题
vue3 watch 监听两个参数
在 Vue 3 中,您可以使用 `watch` 函数来监听多个参数。下面是一个示例代码,演示了如何使用 `watch` 监听两个参数:
```vue
<template>
<div>
<input v-model="firstName" placeholder="First Name" />
<input v-model="lastName" placeholder="Last Name" />
<p>Full Name: {{ fullName }}</p>
</div>
</template>
<script>
import { defineComponent, watch, ref } from 'vue';
export default defineComponent({
name: 'MyComponent',
setup() {
const firstName = ref('');
const lastName = ref('');
watch([firstName, lastName], ([newFirstName, newLastName], [oldFirstName, oldLastName]) => {
console.log('First Name:', newFirstName, 'Last Name:', newLastName);
// 在这里执行您想要的逻辑
});
const fullName = computed(() => `${firstName.value} ${lastName.value}`);
return {
firstName,
lastName,
fullName,
};
},
});
</script>
```
在这个示例中,我们使用了 `watch` 函数来同时监听 `firstName` 和 `lastName` 这两个参数。当其中任何一个参数发生改变时,回调函数将被触发。回调函数接收两个参数,第一个参数是新的值,第二个参数是旧的值。
您可以在回调函数中执行任何您想要的逻辑,例如打印参数的值、执行其他函数等。
希望这个示例对您有所帮助!如果您还有其他问题,请随时提问。
vue3 watch监听多个
在Vue 3中,如果你想在一个`watch`块中监听并处理多个变量的变化,你可以直接在回调函数中声明这些变量作为参数或者通过解构赋值来访问它们。例如:
```js
setup() {
let count = ref(0);
let text = ref('');
const handleChanges = ({ count: newCount, text: newText }) => {
console.log('count changed:', newCount);
console.log('text changed:', newText);
// 更新其他逻辑...
};
watch([count, text], handleChanges);
// 使用方式
count.value++;
text.value = 'New Text';
}
```
在这个例子中,`handleChanges`函数接收一个对象,包含了`count`和`text`的新值。这样,当你修改这两个变量时,`handleChanges`都会被执行。你也可以根据需要选择在对象中给每个变量单独命名,如下:
```js
watch({
count(newCount) {
console.log('count changed:', newCount);
},
text(newText) {
console.log('text changed:', newText);
}
}, () => {
// 如果你想同时处理两个属性,这里不需要传入新的值
});
```
在使用`watch`时,记得它会在组件渲染时初始化,所以在`setup`函数中定义并注册`watch`,并在适当的地方更新变量,以便监听其变化。同时,`watch`会监控所有依赖的变量,所以只要这些变量有所改变,就会触发回调。
阅读全文
相关推荐
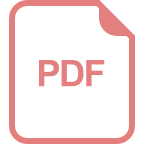
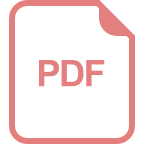













