在springBoot框架为后端,vue框架为前端的共享经济系统毕设项目中考虑使用沙箱环境进行测试,该如何实现,给出代码
时间: 2024-11-11 15:37:07 浏览: 21
在Spring Boot后端与Vue前端的共享经济系统项目中,为了隔离测试环境,特别是涉及到敏感数据或操作时,可以使用沙箱(也称为隔离沙盒或模拟数据库)来创建安全的测试环境。这通常通过以下步骤实现:
1. **选择沙箱库**:
对于前端,可以考虑`vue-test-utils`配合`sandbox-api`这样的库,它允许你在测试环境中模拟API请求。
```javascript
// Vue.js 测试文件
import { createLocalSandbox } from 'sandbox-api';
const sandbox = createLocalSandbox();
beforeEach(() => {
// 清空沙箱或设置初始状态
sandbox.reset();
});
afterEach(() => {
// 关闭沙箱
sandbox.close();
});
// 在测试中使用模拟数据
it('fetches data', async () => {
// 使用sandbox.get()模拟API响应
await sandbox.get('/api/products').then(response => {
expect(response.data).toEqual([]);
});
});
```
2. **后端Spring Boot方面**:
可以使用`MockMvc`来创建模拟的RESTful API服务,对于HTTP请求进行预定义的响应。例如:
```java
// Spring Boot测试类
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
@Autowired
private MockMvc mockMvc;
@MockBean
private ProductService productService;
@Test
public void testFetchProducts() throws Exception {
when(productService.fetchProducts()).thenReturn(List.of(...)); // 模拟产品列表
mockMvc.perform(MockMvcRequestBuilders.get("/api/products")
.contentType(MediaType.APPLICATION_JSON))
.andExpect(status().isOk())
.andExpect(jsonPath("$", hasSize(2))) // 预期返回2个产品
.andExpect(jsonPath("$[0].name", is("Product 1"))); // 断言第一个产品的名称
}
```
在这个例子中,你需要在测试前配置好`MockMvc`并设置mock对象的行为。当真实应用访问这些路径时,会直接使用预定义好的响应。
阅读全文
相关推荐
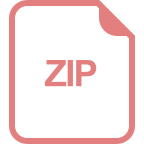
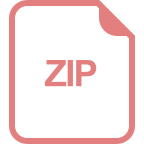
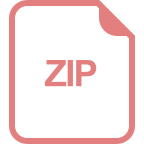
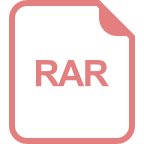
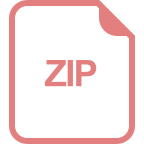
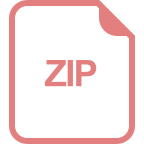
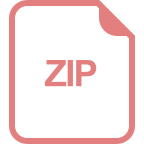
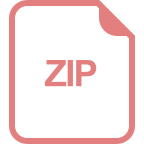
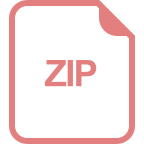
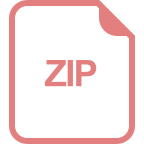
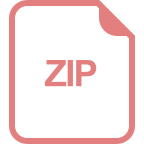
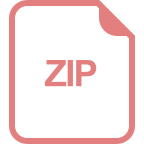
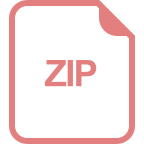
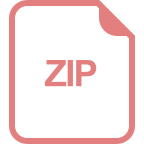
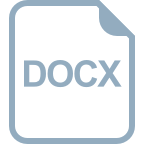