如何使用Python的面向对象方法实现图像的四则运算,并给出具体的代码实例?
时间: 2024-11-18 18:21:46 浏览: 0
在面向对象编程中,实现图像的四则运算需要定义一个图像类,然后在该类中封装加、减、乘、除运算的逻辑。以Python为例,你可以利用PIL库处理图像文件,利用numpy库进行高效的矩阵运算。以下是具体实现的步骤:
参考资源链接:[Python面向对象编程:图像四则运算实战](https://wenku.csdn.net/doc/73ih5vm4aq?spm=1055.2569.3001.10343)
1. 首先,需要安装并导入PIL库和numpy库:
```python
from PIL import Image
import numpy as np
```
2. 定义一个图像类`ImageObject`,用于封装图像操作:
```python
class ImageObject:
def __init__(self, path):
self.image = Image.open(path)
self.array = np.array(self.image)
def __add__(self, other):
result = np.add(self.array, other.array)
result = np.clip(result, 0, 255).astype(np.uint8)
return ImageObject.fromarray(result)
def __sub__(self, other):
result = np.subtract(self.array, other.array)
result = np.clip(result, 0, 255).astype(np.uint8)
return ImageObject.fromarray(result)
def __mul__(self, factor):
result = np.multiply(self.array, factor)
result = np.clip(result, 0, 255).astype(np.uint8)
return ImageObject.fromarray(result)
def __truediv__(self, factor):
result = np.divide(self.array, factor)
result = np.clip(result, 0, 255).astype(np.uint8)
return ImageObject.fromarray(result)
@staticmethod
def fromarray(array):
return ImageObject(np.asarray(Image.fromarray(array)))
```
3. 使用类的实例进行图像的四则运算:
```python
img1 = ImageObject('path_to_image1.jpg')
img2 = ImageObject('path_to_image2.jpg')
result_add = img1 + img2 # 图像加法
result_sub = img1 - img2 # 图像减法
result_mul = img1 * 2 # 图像乘以数字
result_div = img1 / 2 # 图像除以数字
```
在上述代码中,`__add__`和`__sub__`方法实现了两个图像对象之间的加减运算,而`__mul__`和`__truediv__`方法实现了图像对象与数字的乘除运算。注意,运算结果需要使用`np.clip`来确保像素值在0到255的范围内,并转换为合适的numpy数组类型,以便能够用PIL库将numpy数组转换回图像。
通过这种方式,你可以方便地利用面向对象的方法处理图像的四则运算,使得代码更加模块化和易于维护。学习《Python面向对象编程:图像四则运算实战》可以深入了解这些概念,并通过实战提高图像处理的技能。
参考资源链接:[Python面向对象编程:图像四则运算实战](https://wenku.csdn.net/doc/73ih5vm4aq?spm=1055.2569.3001.10343)
阅读全文
相关推荐
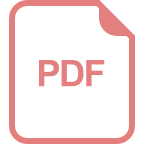
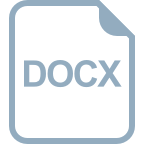
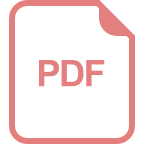
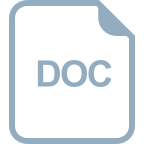

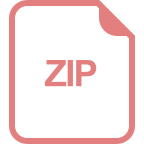
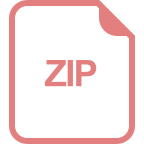
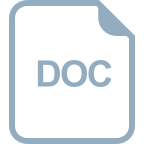
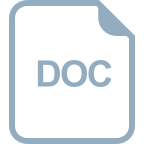
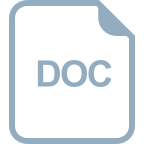
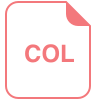
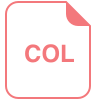
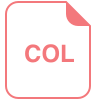
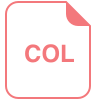
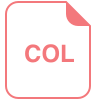
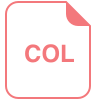
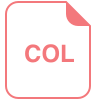
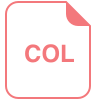
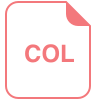