用android实现蓝牙模块功能,查询附近蓝牙设备,点击连接,可以显示连接是否成功等,给出完整代码包括布局
时间: 2024-12-24 07:27:18 浏览: 0
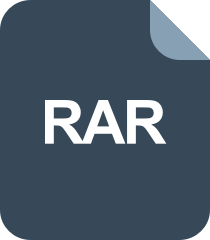
Xinche.rar_android 小车_android 蓝牙_蓝牙_蓝牙 串口 源代码_蓝牙串口android
在Android中实现蓝牙功能涉及多个部分,包括蓝牙适配器、设备搜索以及连接管理。以下是一个简单的示例,展示了如何在用户界面中搜索并连接蓝牙设备,并展示连接状态:
首先,我们需要在XML布局文件(例如activity_main.xml)中包含一个按钮和一个TextView用于显示连接状态:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/search_bluetooth_button"
android:text="搜索蓝牙设备"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onSearchButtonClick"/>
<TextView
android:id="@+id/connect_status_textview"
android:text="未连接"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"/>
</LinearLayout>
```
然后,在对应的Activity中编写Java代码:
```java
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Button searchButton;
private TextView connectStatusTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
searchButton = findViewById(R.id.search_bluetooth_button);
connectStatusTextView = findViewById(R.id.connect_status_textview);
searchButton.setOnClickListener(this);
}
@Override
public void onClick(View v) {
if (v.getId() == R.id.search_bluetooth_button) {
Intent discoverableIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_DISCOVERABLE);
discoverableIntent.putExtra(BluetoothAdapter.EXTRA_DISCOVERABLE_DURATION, 300); // 设置可见时间300秒
startActivityForResult(discoverableIntent, REQUEST_CODE_SEARCH);
connectStatusTextView.setVisibility(View.VISIBLE);
searchButton.setText("已搜索");
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_CODE_SEARCH && resultCode == RESULT_OK) {
BluetoothAdapter adapter = BluetoothAdapter.getDefaultAdapter();
if (adapter != null) {
// 开始扫描附近的蓝牙设备
adapter.startDiscovery();
} else {
Toast.makeText(this, "蓝牙功能不可用", Toast.LENGTH_SHORT).show();
}
}
}
// 连接蓝牙设备的逻辑在此处编写(需要自定义BluetoothDeviceConnection类)
private static final int REQUEST_CODE_SEARCH = 1;
}
```
注意,上面的代码只是一个基础框架,实际的蓝牙连接涉及到复杂的错误处理、设备选择、连接请求以及数据通信。这部分代码需要你自己填充蓝牙连接的具体实现,这通常包括创建`BluetoothDevice`对象、创建`BluetoothSocket`进行连接等。
阅读全文
相关推荐
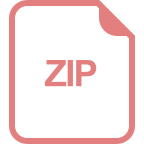
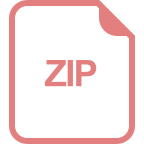
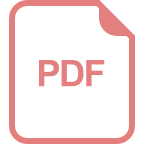
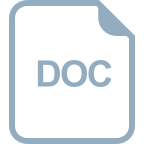
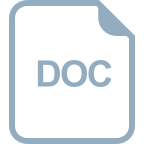
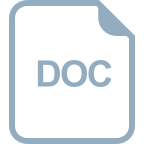
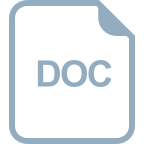



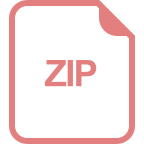
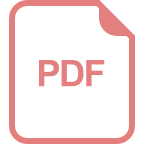
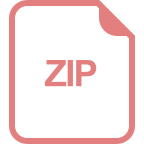
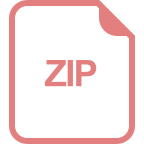
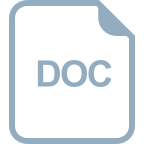
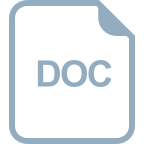
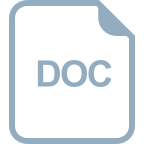