使用 vertx进行mqtt 开发 spring boot整合vertx开发mqtt
时间: 2023-08-01 17:09:18 浏览: 112
要在Spring Boot中整合Vert.x开发MQTT应用程序,可以按照以下步骤进行操作:
1. 添加依赖:在项目的pom.xml文件中添加以下依赖,包括Vert.x和MQTT相关的依赖:
```xml
<dependency>
<groupId>io.vertx</groupId>
<artifactId>vertx-spring-boot-starter</artifactId>
<version>2.1.6</version>
</dependency>
<dependency>
<groupId>io.vertx</groupId>
<artifactId>vertx-mqtt-server</artifactId>
<version>4.2.1</version>
</dependency>
```
2. 创建MQTT服务器:在Spring Boot应用程序的入口类中,创建一个Vert.x的`Vertx`实例,并使用`Vertx`实例创建一个`MqttServer`实例。
```java
import io.vertx.core.Vertx;
import io.vertx.mqtt.MqttServer;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Bean
public MqttServer mqttServer(Vertx vertx) {
return MqttServer.create(vertx);
}
}
```
3. 编写MQTT消息处理器:创建一个实现`MqttEndpointHandler`接口的类,用于处理接收到的MQTT消息。可以根据业务需求自定义消息处理逻辑。
```java
import io.vertx.mqtt.MqttEndpoint;
import io.vertx.mqtt.MqttEndpointHandler;
public class MyMqttEndpointHandler implements MqttEndpointHandler {
@Override
public void handle(MqttEndpoint endpoint) {
// 处理消息逻辑
}
}
```
4. 注册MQTT消息处理器:在Spring Boot应用程序的入口类中,将自定义的`MqttEndpointHandler`实例注册到`MqttServer`中。
```java
import io.vertx.core.Vertx;
import io.vertx.mqtt.MqttServer;
import io.vertx.mqtt.MqttServerOptions;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Bean
public MqttServer mqttServer(Vertx vertx, MqttEndpointHandler endpointHandler) {
MqttServerOptions options = new MqttServerOptions()
.setPort(1883); // 设置MQTT服务器端口
MqttServer mqttServer = MqttServer.create(vertx, options);
mqttServer.endpointHandler(endpointHandler); // 注册消息处理器
return mqttServer;
}
@Bean
public MqttEndpointHandler endpointHandler() {
return new MyMqttEndpointHandler();
}
}
```
通过以上步骤,你就可以在Spring Boot应用程序中使用Vert.x开发MQTT应用程序了。启动应用程序后,Vert.x MQTT服务器将在指定的端口上监听并处理接收到的MQTT消息。你可以根据需要在`MyMqttEndpointHandler`中编写自定义的消息处理逻辑。
相关推荐
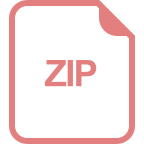
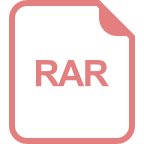














