在Linux环境下,如何编写一个使用ICMP Echo请求的主机存活检测脚本,并说明其校验和计算过程?
时间: 2024-11-07 20:20:19 浏览: 18
为了有效地检测网络中主机的存在性,你可以通过编写一个脚本来实现基于ICMP Echo请求的主机存活检测。在Linux环境下,可以使用原始套接字来构建和发送ICMP Echo请求,并监听目标主机的Echo Reply响应。以下是实现这一功能的详细步骤和代码示例:
参考资源链接:[利用ICMP协议进行主机扫描:原理与Linux实现](https://wenku.csdn.net/doc/5u7ovp38gi?spm=1055.2569.3001.10343)
首先,你需要了解ICMP数据包的IP头部和ICMP头部结构,以便正确构建和发送ICMP Echo请求。ICMP Echo请求类型为8,而Echo Reply类型为0。每个ICMP数据包都包含一个校验和字段,用于检验数据的完整性。
在Linux中,可以使用`socket`和`bind`系统调用来创建原始套接字,并通过`sendto`函数发送自定义的ICMP Echo请求。为了监听Echo Reply,你需要使用`recvfrom`函数。
在发送和接收数据包时,必须填充IP头部和ICMP头部的各个字段,如校验和。校验和的计算方法包括将数据包中的所有16位字相加,然后将这个总和的反码作为校验和填入数据包。
下面是一个简单的示例代码,用于构建和发送ICMP Echo请求,以及计算校验和的过程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <netinet/ip_icmp.h>
#include <netinet/udp.h>
#include <netinet/tcp.h>
#include <netinet/ip.h>
#include <arpa/inet.h>
#include <sys/socket.h>
// 计算校验和的函数
unsigned short calculate_checksum(void *b, int len) {
unsigned short *buf = b;
unsigned int sum = 0;
unsigned short result;
for (sum = 0; len > 1; len -= 2) {
sum += *buf++;
}
if (len == 1) {
sum += *(unsigned char *)buf;
}
sum = (sum >> 16) + (sum & 0xFFFF);
sum += (sum >> 16);
result = ~sum;
return result;
}
// 主函数
int main(int argc, char *argv[]) {
int sockfd;
int ttl = 64;
struct sockaddr_in dest_info;
char send_buf[1500], recv_buf[1500];
int send_buf_len;
struct iphdr *ip;
struct icmp *icmp;
char msg[1024];
// 创建原始套接字
sockfd = socket(AF_INET, SOCK_RAW, IPPROTO_ICMP);
// 填充IP头部和ICMP头部
// ...
// 计算校验和并填充到数据包中
// ...
// 发送ICMP Echo请求
sendto(sockfd, send_buf, send_buf_len, 0, (struct sockaddr*)&dest_info, sizeof(dest_info));
// 接收Echo Reply
recvfrom(sockfd, recv_buf, 1500, 0, NULL, NULL);
// 关闭套接字
close(sockfd);
return 0;
}
```
在上述代码中,你需要根据实际情况填充IP头部和ICMP头部的各个字段,并计算校验和。接收函数`recvfrom`将等待目标主机的Echo Reply响应。如果收到响应,表示目标主机是可达的,否则可能表示目标主机不可达或存在防火墙阻止ICMP包。
为了获得更深入的理解和更多的编程细节,建议深入阅读资料《利用ICMP协议进行主机扫描:原理与Linux实现》。这本书提供了详细的技术讲解和代码示例,将帮助你全面掌握ICMP协议在主机存活检测中的应用,并能够在网络安全领域进一步扩展知识。
参考资源链接:[利用ICMP协议进行主机扫描:原理与Linux实现](https://wenku.csdn.net/doc/5u7ovp38gi?spm=1055.2569.3001.10343)
阅读全文
相关推荐
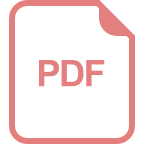
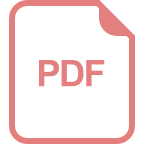
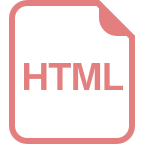

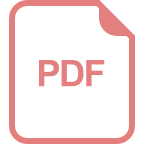
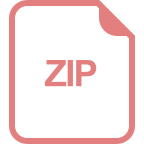
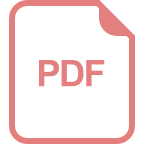
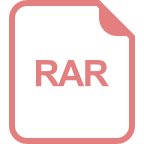
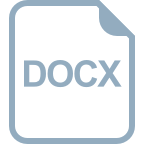
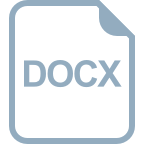
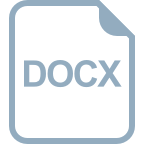
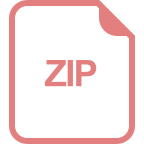
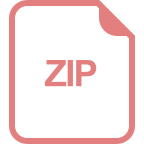
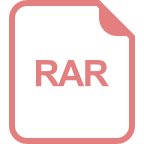
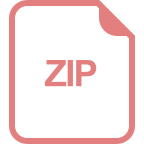
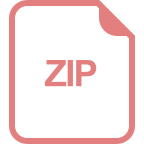
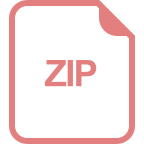