springboot使用注解开发mybatis
时间: 2023-10-31 09:19:43 浏览: 97
对于使用注解开发MyBatis的Spring Boot项目,你可以按以下步骤进行操作:
1. 首先,在Spring Boot项目中添加MyBatis和MyBatis-Spring的依赖,可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
```
2. 创建一个数据源配置类,用于配置数据库连接信息,例如:
```java
@Configuration
@MapperScan(basePackages = "com.example.mapper")
public class DataSourceConfig {
@Value("${spring.datasource.url}")
private String url;
@Value("${spring.datasource.username}")
private String username;
@Value("${spring.datasource.password}")
private String password;
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl(url);
dataSource.setUsername(username);
dataSource.setPassword(password);
return dataSource;
}
}
```
3. 创建Mapper接口,并使用@Mapper注解标识该接口为MyBatis的Mapper接口,例如:
```java
@Mapper
public interface UserMapper {
@Select("SELECT * FROM user")
List<User> getAllUsers();
// 其他CRUD方法
}
```
4. 在application.properties或application.yml文件中配置数据库连接信息,例如:
```yaml
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: root
password: password
```
5. 在Spring Boot的启动类上添加@MapperScan注解,指定要扫描的Mapper接口所在的包,例如:
```java
@SpringBootApplication
@MapperScan("com.example.mapper")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
这样,你就可以在Spring Boot项目中使用注解开发MyBatis了。通过在Mapper接口的方法上使用注解来实现CRUD操作,Spring Boot会自动扫描并注册这些Mapper接口的实现,无需手动编写映射文件。
阅读全文
相关推荐
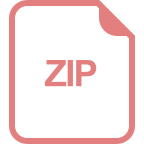
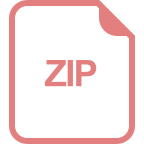
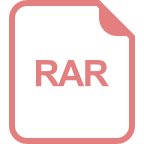
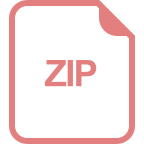
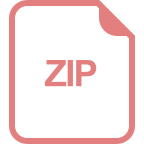
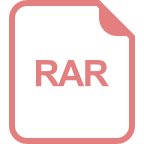
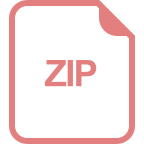
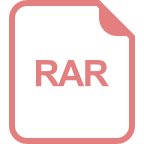
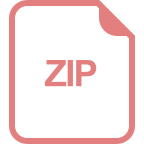
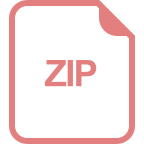
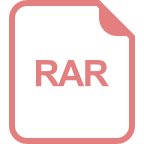
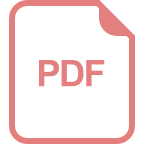
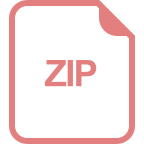
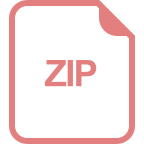
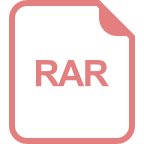
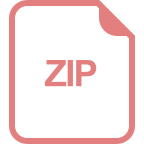