编写c语言程序,用for语句,随机输入15个整数,可以是正数、负数和零,计算它们的和、所有正数之和及所有负数之和,分类统计正数、负数以及零的个数,并分别打印出来。
时间: 2024-11-20 13:39:04 浏览: 10
当你需要编写一个C语言程序来完成这个任务时,你可以按照以下步骤操作:
1. 首先,导入必要的头文件`<stdio.h>`和`<stdlib.h>`,前者用于标准输入输出,后者包含生成随机数的函数。
```c
#include <stdio.h>
#include <stdlib.h>
```
2. 使用`for`循环来读取15个整数,并检查每个数的正负性。这里会创建三个计数器(一个总和,一个正数和一个负数),以及一个临时变量来存储当前输入的值。
```c
int total = 0, positive = 0, negative = 0;
int input, temp;
for (int i = 0; i < 15; i++) {
printf("请输入第 %d 个整数:", i + 1);
scanf("%d", &input);
temp = input;
if (temp > 0) {
positive++;
total += temp;
} else if (temp < 0) {
negative++;
total -= temp;
}
}
```
3. 计算完所有数值之后,分别打印出总数、正数之和、负数之和以及各自的数量。
```c
printf("\n总的和:%d\n", total);
printf("正数之和:%d\n", positive * (positive == 0 ? 0 : positive));
printf("负数之和:%d\n", negative * (negative == 0 ? 0 : -negative));
printf("零的数量:%d\n", 15 - positive - negative); // 注意这里是15减去非零数的计数
getchar(); // 添加此行是为了防止缓冲区溢出
```
完整的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
int total = 0, positive = 0, negative = 0;
int input, temp;
for (int i = 0; i < 15; i++) {
printf("请输入第 %d 个整数:", i + 1);
scanf("%d", &input);
temp = input;
if (temp > 0) {
positive++;
total += temp;
} else if (temp < 0) {
negative++;
total -= temp;
}
}
printf("\n总的和:%d\n", total);
printf("正数之和:%d\n", positive * (positive == 0 ? 0 : positive));
printf("负数之和:%d\n", negative * (negative == 0 ? 0 : - negative);
getchar();
return 0;
}
```
阅读全文
相关推荐
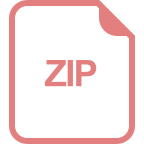
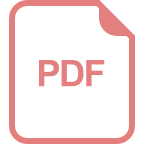
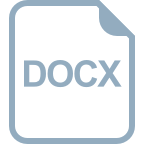















