C#如何读取程序内存
时间: 2024-09-13 14:11:20 浏览: 39
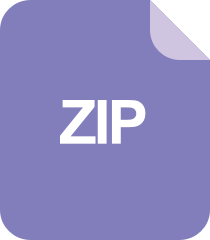
c#编写的内存读取修改器

在C#中,读取程序内存通常涉及到使用反射、指针操作、P/Invoke调用本地API等技术。下面是一些常用的方法:
1. 反射(Reflection):可以用来访问程序集中的类型、成员、模块等信息,也能够读取和修改对象的字段值。使用反射时,可以通过对象的Type对象来获取对象的字段信息,然后读取字段的值。
```csharp
using System;
using System.Reflection;
class Program
{
static void Main()
{
ExampleClass obj = new ExampleClass();
Type type = obj.GetType();
PropertyInfo propInfo = type.GetProperty("ExampleProperty");
Console.WriteLine(propInfo.GetValue(obj, null));
}
}
public class ExampleClass
{
public int ExampleProperty { get; set; } = 123;
}
```
2. 指针操作:虽然C#是一种安全的编程语言,但是通过unsafe代码块和指针,依然可以进行底层内存的读取和写入。这需要程序被编译为允许不安全代码。
```csharp
using System;
class Program
{
static void Main()
{
int number = 123;
unsafe
{
int* pNumber = &number;
Console.WriteLine(*pNumber);
}
}
}
```
3. P/Invoke调用本地API:C#可以通过P/Invoke机制调用Windows API或其他本地库中的函数,用于读取内存、获取进程信息等。
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("kernel32.dll")]
public static extern IntPtr OpenProcess(int dwDesiredAccess, bool bInheritHandle, int dwProcessId);
[DllImport("kernel32.dll", SetLastError=true)]
public static extern bool ReadProcessMemory(IntPtr hProcess, IntPtr lpBaseAddress, byte[] lpBuffer, int dwSize, out IntPtr lpNumberOfBytesRead);
static void Main()
{
IntPtr hProcess = OpenProcess(0x1F0FFF, false, GetCurrentProcessId());
// 读取内存操作...
}
}
```
需要注意的是,直接操作内存是非常危险的,因为错误的内存操作可能导致程序崩溃、数据损坏甚至安全漏洞。因此,除非必要,否则不建议在生产环境中直接读取程序内存。此外,上述代码只是演示了如何使用这些技术,并没有实现具体的内存读取功能,因为这通常需要对目标程序的内存结构有深入的了解。
阅读全文
相关推荐
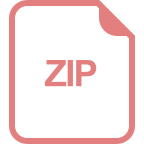
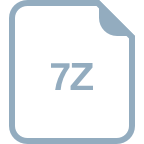

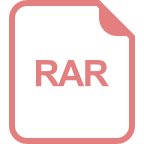
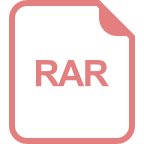

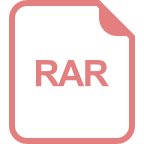
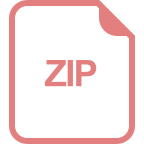
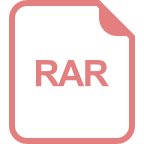
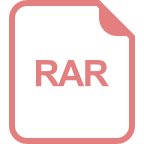
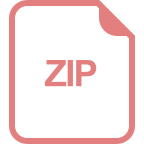
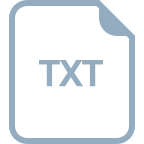
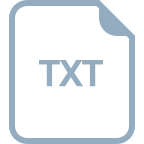
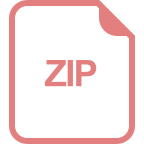
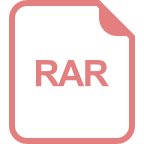
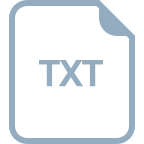
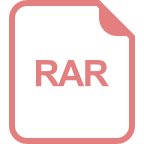
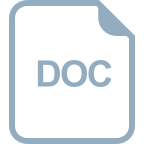