完整 用c++写一个求解一元二次方程的代码 要求函数利用引用传参
时间: 2024-09-08 20:03:37 浏览: 77
为了实现一个完整的C++程序,按照题目要求,我们将创建四个函数:`get_coefficients`(获取系数),`solve_quadratic`(求解一元二次方程),`trig_solutions`(处理可能的复数解,这里假设仅考虑实数解)以及`display_results`(输出结果)。我们使用引用参数以避免不必要的复制。
```cpp
#include <iostream>
#include <cmath>
// 函数声明,接受引用作为参数
void get_coefficients(double& a, double& b, double& c); // 获取系数
double solve_quadratic(double a, double b, double c); // 求解一元二次方程
void trig_solutions(double x_real, double x_imaginary); // 处理复数解
void display_results(double root1, double root2); // 结果输出
int main() {
double a, b, c; // 全局变量存储系数
get_coefficients(a, b, c);
double real_root1 = solve_quadratic(a, b, c);
double real_root2 = solve_quadratic(-a, -b, c); // 对于实数解,x1 = -b + sqrt(b²-4ac), x2 = -b - sqrt(b²-4ac)
if (std::abs(real_root1 * real_root2) > 1e-6) { // 判断是否接近零,防止浮点误差影响
trig_solutions(real_root1, 0.0); // 只有一个实根时,无需处理虚部
} else {
trig_solutions(real_root1, std::sqrt(std::max(0., -(b*b - 4*a*c)))); // 复数根
}
display_results(real_root1, real_root2);
return 0;
}
// 函数定义
void get_coefficients(double& a, double& b, double& c) {
std::cout << "Enter coefficients a, b, and c for the quadratic equation: ";
std::cin >> a >> b >> c;
}
double solve_quadratic(double a, double b, double c) {
double discriminant = b * b - 4 * a * c;
return (-b + std::sqrt(discriminant)) / (2 * a);
}
void trig_solutions(double x_real, double x_imaginary) {
if (x_imaginary != 0) {
std::cout << "The roots are complex conjugates: (" << x_real << ", " << -x_imaginary << ") and (" << x_real << ", " << x_imaginary << ").";
} else {
std::cout << "The root is real: " << x_real << ".";
}
}
void display_results(double root1, double root2) {
if (root1 == root2) {
std::cout << "Root: " << root1 << std::endl;
} else {
std::cout << "Roots: " << root1 << " and " << root2 << std::endl;
}
}
```
阅读全文
相关推荐


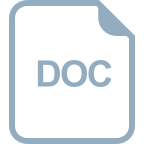












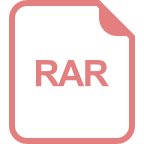


