1.先类型声明和定义头结点,建立一个空的单链表L; I 2.在单链表L的第i个元素之后插入新的元素e; 3.在单链表L中删除第i个元素,并用e返回其值。用c语言版的数据结构写该代码
时间: 2024-10-14 07:12:15 浏览: 41
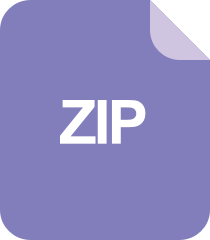
链表-使用C语言实现带头结点的单链表.zip
在C语言中,你可以使用结构体来表示链表节点,并使用指针来操作链表。这里是一个简单的例子,展示如何按照您的需求完成单链表的操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 类型声明并创建空链表
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node)); // 创建头节点
head->data = 0;
head->next = NULL; // 初始化next为空
return head;
}
// 插入新元素到链表的第i个位置
void insertAfterNode(Node** list, int i, int e) {
if (*list == NULL) {
printf("List is empty.\n");
return;
}
Node* current = *list;
for (int j = 0; j < i && current != NULL; j++) {
current = current->next;
}
if (current == NULL) {
printf("Invalid index.\n");
} else {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = e;
newNode->next = current->next;
current->next = newNode;
}
}
// 删除第i个元素并返回其值
int deleteAndReturn(int* list, int i) {
if (*list == NULL) {
printf("List is empty.\n");
return -1; // 或者抛出错误
}
Node* current = *list;
Node* prev = NULL;
for (int j = 0; j < i && current != NULL; j++) {
prev = current;
current = current->next;
}
if (current == NULL) {
printf("Invalid index or empty list after element %d.\n", i);
return -1; // 或者抛出错误
}
int value = current->data;
if (prev != NULL) {
prev->next = current->next;
} else {
*list = current->next; // 如果删除的是头节点
}
free(current); // 释放内存
return value;
}
// 示例用法
int main() {
Node* L = createList();
// ... (后续对链表进行其他操作)
// 插入元素
insertAfterNode(&L, 1, 5);
// 删除元素并打印其值
int deletedValue = deleteAndReturn(&L, 1);
// 现在处理删除后的链表...
return 0;
}
```
阅读全文
相关推荐
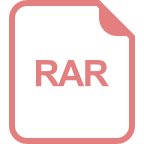
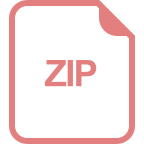















