C语言网络聊天室采用多进程实现私聊功能服务器端代码
时间: 2024-09-25 10:17:28 浏览: 48
C语言在网络聊天室中采用多进程实现私聊功能,通常会利用`fork()`函数创建新进程来模拟。服务器端代码的一个基本框架可能会像这样:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
// 假设有个全局的客户端列表结构
typedef struct Client {
char *username;
int pid; // 子进程PID
} Client;
void handle_client(Client* client) {
while (true) {
char message[1024];
if (fgets(message, sizeof(message), stdin) == NULL) { // 用户输入结束
break;
}
// 发送消息到指定子进程(client.pid)
write(client->pid, message, strlen(message));
}
// 清理子进程资源
close(client->pid);
wait(NULL); // 等待子进程退出
free(client->username);
}
int main() {
// 初始化服务器和接受连接
// 创建一个新的Client结构并为其分配内存
Client new_client;
new_client.username = strdup("用户名"); // 假设用户输入的用户名
new_client.pid = fork(); // 创建新进程
if (new_client.pid == 0) { // 子进程
handle_client(&new_client);
exit(0);
} else if (new_client.pid > 0) { // 主进程
// 将新的子进程加入到客户端列表,并监听其输出
// 当子进程结束,从列表中移除并释放资源
// ... (这里省略管理子进程的代码)
} else {
perror("Fork failed");
return 1;
}
return 0;
}
```
阅读全文
相关推荐










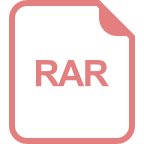







