保存图片到指定文件夹
时间: 2025-01-03 16:40:13 浏览: 13
### 使用Python中的Pillow库保存图像到指定文件夹
对于Python而言,`PIL.Image.save()` 方法允许将图像对象保存至磁盘上的特定位置。为了确保跨平台兼容性,在构建目标路径字符串时应考虑不同操作系统间的差异。
在Windows上,路径分隔符为反斜杠 `\`;而在Linux和macOS中,则采用正斜杠 `/` 或者双反斜杠 `\\` 来表示子目录之间的关系。推荐使用 `os.path.join()` 函数来动态组合路径组件,从而提高代码的可移植性和健壮性[^1]。
下面是一个简单的例子展示如何利用Python把一张图片存入给定的目标文件夹:
```python
from PIL import Image
import os
def save_image_to_folder(image_path, output_folder):
try:
img = Image.open(image_path)
# 创建输出文件夹如果它不存在的话
if not os.path.exists(output_folder):
os.makedirs(output_folder)
# 构建完整的输出路径并保存图像
base_name = os.path.basename(image_path)
full_output_path = os.path.join(output_folder, base_name)
img.save(full_output_path)
print(f"Image saved successfully at {full_output_path}")
except Exception as e:
print(e)
# 调用函数实例化过程
image_file = "example.jpg"
destination_dir = "./output_images/"
save_image_to_folder(image_file, destination_dir)
```
这段脚本首先尝试打开输入参数所指明的源图像文件,接着检查目的文件夹是否存在——如果不存就会自动创建该文件夹。最后一步则是通过调用 `img.save()` 把处理过的图像写入由 `output_folder` 和原始文件名共同构成的新地址里。
#### Java 中保存图像到指定文件夹的方式
当涉及到Java语言时,可以通过BufferedImage类配合ImageIO.write()静态方法完成相似的任务。同样需要注意的是,应当适当地拼接出适用于当前系统的绝对路径。
这里给出一段基本示范代码片段用于说明这一流程:
```java
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class SaveImageExample {
public static void main(String[] args){
String inputImagePath = "/path/to/source/image.png";
File inputFile = new File(inputImagePath);
BufferedImage image = null;
try{
image = ImageIO.read(inputFile);
// 定义输出文件夹的位置以及最终要保存的文件全路径
String targetDirPath = "/desired/output/directory/";
File dir = new File(targetDirPath);
if(!dir.exists()){
dir.mkdirs();
}
String fileName = "copied-image.png";
File outputFile = new File(dir.getAbsolutePath(), fileName);
// 将缓冲区内的图像数据写出成PNG格式的文件
boolean result = ImageIO.write(image,"png",outputFile);
System.out.println(result ? "成功保存":"失败");
}catch(IOException ex){
ex.printStackTrace();
}
}
}
```
此段程序先加载了一张名为`image.png`的位图资源作为工作副本,之后按照上述逻辑判断是否有必要建立新的存储空间,并且执行实际的数据流写入动作以形成物理文件[^4]。
阅读全文
相关推荐









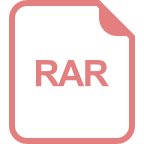







