获取yolov8预测类别
时间: 2024-07-23 12:01:08 浏览: 173
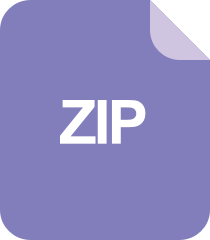
YOLOV8分类预训练模型
YOLOv8(You Only Look Once Version 8)是一种流行的物体检测算法,它通常用于实时场景中的目标检测任务。在完成图像分类后,YOLOv8会返回每个检测区域的信息,包括位置坐标和置信度得分。预测类别通常是通过模型内部的概率分布来确定的,这个概率反映了该区域对应各类别的可能性。
获取YOLOv8预测类别的步骤通常如下:
1. **前向传播**:将输入图像送入训练好的YOLOv8神经网络模型。
2. **解码**:模型会输出一组包含边界框和预测分数的网格,需要对这些结果进行解码,找出每个检测到的目标的位置。
3. **非极大抑制(NMS)**:为了去除重复的预测,应用非极大值抑制来选择最有可能的对象。
4. **类别识别**:从每个预测边界框对应的置信度得分最高的类别作为预测类别。
如果你在Python环境中使用YOLOv8库(如`pytorch-yolov5`或`ultralytics.yolov8`),可以按照库文档提供的API直接访问这些信息。例如,在`ultralytics.yolov8`中,你可以使用`model.predict()`得到`Prediction`对象,其中包含了`class`属性表示预测的类别。
阅读全文
相关推荐
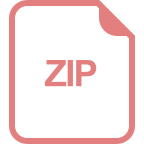
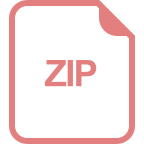
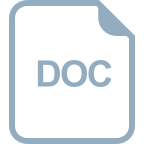














