将单链表逆转用C++
时间: 2024-10-12 20:15:27 浏览: 29
在C++中,反转一个单链表可以使用迭代或递归的方式来实现。以下是使用迭代方法的一个示例:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
// 反转链表的函数
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr; // 前驱指针,初始为NULL
ListNode* current = head; // 当前指针,初始指向头结点
while (current != nullptr) { // 当有元素时
ListNode* temp = current->next; // 保存当前节点的下一个节点
current->next = prev; // 更新当前节点的next指向前一个节点
prev = current; // 预先指针前进到当前节点
current = temp; // 当前节点移动到下一个节点
}
return prev; // 返回新的头结点(原尾部现在成为新头部)
}
int main() {
// 示例:创建链表 1 -> 2 -> 3 -> 4 -> 5
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(4);
head->next->next->next->next = new ListNode(5);
std::cout << "Original List: ";
printList(head); // 打印原始链表
ListNode* reversedHead = reverseList(head);
std::cout << "\nReversed List: ";
printList(reversedHead); // 打印反转后的链表
return 0;
}
// 辅助函数打印链表
void printList(ListNode* node) {
while (node != nullptr) {
std::cout << node->val << " ";
node = node->next;
}
std::cout << "\n";
}
阅读全文
相关推荐
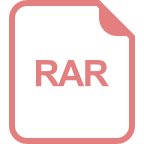
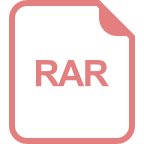














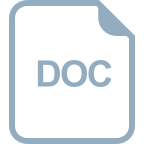
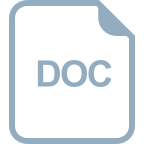