javaweb添加一个实现增删改的控制器
时间: 2024-10-23 15:04:18 浏览: 22
在Java Web中,添加一个支持增删改操作的控制器通常涉及以下几个步骤:
1. **创建Controller类**:
首先,你需要创建一个`@RestController`注解的类,因为这是Spring MVC用于处理HTTP请求的控制器。例如:
```java
@RestController
public class MyOperationsController {
//...
}
```
2. **CRUD操作方法**:
- **新增(Create)**:使用`@PostMapping`接收POST请求并保存数据。可以使用`@RequestBody`来自动绑定请求体到方法参数。
```java
@PostMapping("/add")
public ResponseEntity<?> add(@Valid @RequestBody YourModel model) {
yourService.save(model); //假设yourService是一个处理业务逻辑的服务
return ResponseEntity.ok().build();
}
```
- **读取(Read)**:使用`@GetMapping`处理GET请求,查询数据库。
```java
@GetMapping("/read/{id}")
public ResponseEntity<YourModel> read(@PathVariable Long id) {
YourModel model = yourService.findById(id);
if (model == null) {
return new ResponseEntity<>(HttpStatus.NOT_FOUND);
}
return ResponseEntity.ok(model);
}
```
- **更新(Update)**:使用`@PutMapping`或`@PatchMapping`处理PUT或PATCH请求,更新已有数据。
```java
@PutMapping("/update/{id}")
public ResponseEntity<?> update(@PathVariable Long id, @Valid @RequestBody YourModel updatedModel) {
updatedModel.setId(id); //假设更新ID不能改变
yourService.update(updatedModel);
return ResponseEntity.ok().build();
}
```
- **删除(Delete)**:使用`@DeleteMapping`处理DELETE请求,移除指定的数据。
```java
@DeleteMapping("/delete/{id}")
public ResponseEntity<?> delete(@PathVariable Long id) {
yourService.deleteById(id);
return ResponseEntity.noContent().build();
}
```
3. **错误处理和异常映射**:
异常处理是必要的,可以使用`@ExceptionHandler`处理可能出现的异常,并返回合适的HTTP状态码。
4. **依赖注入和服务层分离**:
`yourService`应该是通过构造函数或者setter注入的方式,以便于单元测试和依赖管理。
记得在实际应用中,你需要将`YourModel`、`yourService`等替换为具体的模型类和业务服务。
阅读全文
相关推荐










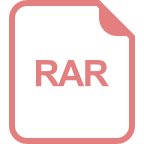






