nestjs typeorm 怎么做回滚事务
时间: 2024-03-01 22:48:44 浏览: 242
在NestJS中使用TypeORM进行事务回滚可以通过以下步骤实现:
1. 首先,确保你已经安装了NestJS和TypeORM,并且已经设置好了数据库连接。
2. 在你的服务类中,导入TypeORM的相关模块和装饰器:
```typescript
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository, Connection } from 'typeorm';
```
3. 在你的服务类中,注入TypeORM的Repository和Connection:
```typescript
@Injectable()
export class YourService {
constructor(
@InjectRepository(YourEntity)
private readonly yourRepository: Repository<YourEntity>,
private readonly connection: Connection,
) {}
}
```
4. 在你的服务类中,创建一个方法来执行事务操作:
```typescript
async yourTransactionMethod(): Promise<void> {
const queryRunner = this.connection.createQueryRunner();
await queryRunner.connect();
await queryRunner.startTransaction();
try {
// 在这里执行你的数据库操作
// 例如:await queryRunner.manager.save(yourEntity);
await queryRunner.commitTransaction();
} catch (error) {
await queryRunner.rollbackTransaction();
} finally {
await queryRunner.release();
}
}
```
在上述代码中,我们使用`queryRunner`对象来执行数据库操作。如果操作成功,我们调用`commitTransaction()`方法来提交事务。如果操作失败,我们调用`rollbackTransaction()`方法来回滚事务。最后,我们调用`release()`方法来释放`queryRunner`对象。
5. 在你的控制器中,调用事务方法:
```typescript
@Post()
async yourControllerMethod(): Promise<void> {
await this.yourService.yourTransactionMethod();
}
```
这样,当你调用控制器的方法时,事务将会被执行,并根据操作结果进行提交或回滚。
阅读全文
相关推荐




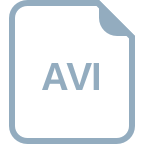










