凯撒加密Python
时间: 2023-11-19 15:50:34 浏览: 90
好的,关于凯撒加密的Python实现,可以使用以下代码:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
if char.isupper():
result += chr((ord(char) + shift - 65) % 26 + 65)
else:
result += chr((ord(char) + shift - 97) % 26 + 97)
else:
result += char
return result
```
其中,`text`是要加密的文本,`shift`是移位数。这个函数会返回加密后的文本。
例如,如果要将文本`hello world`向右移动3个位置,可以这样调用函数:
```python
caesar_cipher("hello world", 3)
```
输出结果为:`khoor zruog`
相关问题
凯撒加密python
凯撒加密是一种简单的替换密码,可以通过将字母按照一定的偏移量进行替换来实现加密。以下是一个使用Python实现凯撒加密的示例代码:
```python
def caesar_encrypt(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha(): # 只对字母进行加密,忽略其他字符
ascii_offset = ord('a') if char.islower() else ord('A')
encrypted_char = chr((ord(char) - ascii_offset + shift) % 26 + ascii_offset)
encrypted_text += encrypted_char
else:
encrypted_text += char
return encrypted_text
# 测试示例
text = "Hello, World!"
shift = 3
encrypted_text = caesar_encrypt(text, shift)
print("加密后的文本:", encrypted_text)
```
这段代码会将字符串 "Hello, World!" 使用偏移量为 3 进行加密,得到加密后的文本 "Khoor, Zruog!"。你可以根据需要修改偏移量和要加密的文本。
凯撒加密Python程序
凯撒加密(Caesar Cipher),也称为移位密码,是一种简单的替换式加密技术。在Python中实现凯撒加密,你可以通过循环遍历字母并按照指定的偏移量进行替换。下面是一个基础的Python程序示例:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
# 对大写字母和小写字母处理不同
shifted = ord(char) + shift
if char.isupper():
new_char = chr(shifted % 26 + 65)
else:
new_char = chr(shifted % 26 + 97)
else:
new_char = char
result += new_char
return result
# 使用示例
plaintext = "Hello, World!"
shift = 3
ciphertext = caesar_cipher(plaintext, shift)
print(f"原文: {plaintext}")
print(f"密文: {ciphertext}")
阅读全文
相关推荐
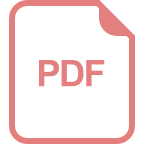
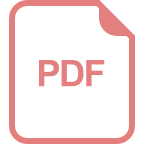













