读取自定义配置文件并生成二维码Springboot代码
时间: 2024-09-26 14:03:12 浏览: 6
在Spring Boot项目中,读取自定义配置文件并生成二维码通常涉及以下几个步骤:
1. **配置文件**:
创建或修改application.properties或application.yml这样的配置文件,可以添加一个用于存储二维码数据的键值对,例如:
```properties
qr-code.data=my_qr_data
```
2. **配置扫描器**:
首先需要引入QRCode库,如`com.github.zbar:jzbar` 或者 `net.glxn.qrgen:qrcodegen`,这取决于你选择的生成二维码的API。
3. **服务类**:
在Service或Controller类中,你可以创建一个方法,利用配置文件的数据生成二维码。假设你使用的是`qrcodegen`库:
```java
@ConfigurationProperties(prefix = "qr-code")
public class QrCodeConfig {
private String data;
// getters and setters...
}
@Service
public class QrCodeGeneratorService {
private final QrCodeConfig config;
public QrCodeGeneratorService(QrCodeConfig config) {
this.config = config;
}
public BufferedImage generateQrCode() throws IOException {
QRCodeWriter writer = new QRCodeWriter();
ByteArrayOutputStream stream = new ByteArrayOutputStream();
writer.encode(config.getData(), BarcodeFormat.QR_CODE, 400, 400); // QR Code size
ImageIO.write(ImageIO.read(new ByteArrayInputStream(stream.toByteArray())), "PNG", null);
return ImageIO.read(new ByteArrayInputStream(stream.toByteArray()));
}
}
```
4. **注入依赖**:
在需要使用二维码的地方,通过@Autowired注解注入`QrCodeGeneratorService`。
5. **调用生成二维码**:
在Controller或某个业务处理方法中,你可以像下面这样调用`generateQrCode()`方法:
```java
@GetMapping("/generate-qr")
public ResponseEntity<byte[]> generateQRCode(QrCodeConfig config) {
try {
BufferedImage qrImage = service.generateQrCode();
byte[] imageBytes = ImageUtils.imageToByteArray(qrImage);
return ResponseEntity.ok().contentType(MediaType.IMAGE_PNG).body(imageBytes);
} catch (IOException e) {
log.error("Failed to generate QR code", e);
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
```
相关推荐
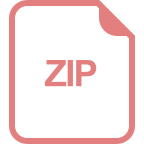
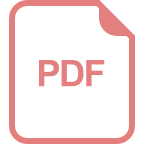
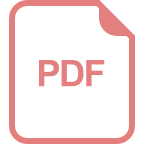
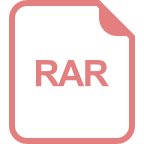
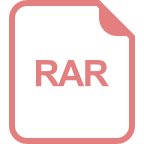
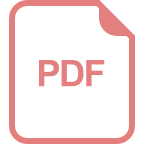
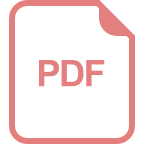
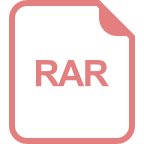
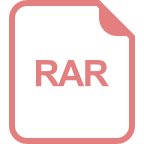
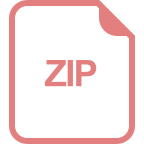
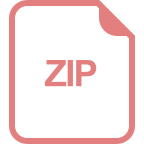
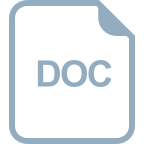
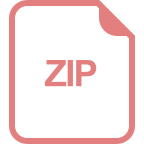
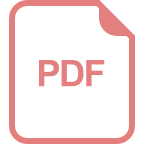
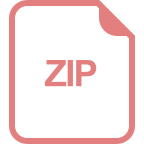
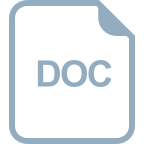
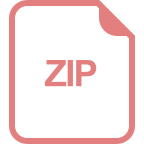
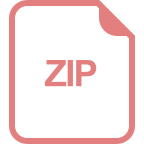
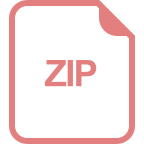