创建一个Rectangle类(矩形类),添加2个属性 width、heiht,添加2个方法计算矩形的周长和面积。编程利用Rectangle类的对象计算并输出一个矩形的周长和面积。
时间: 2024-10-19 21:02:41 浏览: 48
当然可以,下面是一个简单的Python示例,展示了如何创建一个名为Rectangle的类,包含width和height属性以及计算周长和面积的方法:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
# 计算矩形的周长
def perimeter(self):
return 2 * (self.width + self.height)
# 计算矩形的面积
def area(self):
return self.width * self.height
# 使用Rectangle类创建一个实例
rect = Rectangle(5, 4)
# 计算并输出周长
print("矩形的周长:", rect.perimeter())
# 计算并输出面积
print("矩形的面积:", rect.area())
```
在这个例子中,我们首先定义了一个Rectangle类,它有两个构造函数参数`width`和`height`,然后分别定义了`perimeter()`和`area()`方法来计算周长和面积。接着,我们创建了一个Rectangle的实例,并使用这个实例调用了这两个方法。
相关问题
JAVA创建一个Rectangle类(矩形类),添加2个属性 width、heiht,添加2个方法计算矩形的周长和面积。编程利用Rectangle类的对象计算并输出一个矩形的周长和面积。
在Java中,你可以按照以下步骤创建一个Rectangle类来实现这个功能:
```java
// 定义Rectangle类
public class Rectangle {
// 属性
private double width;
private double height;
// 构造函数
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
// 计算周长的方法
public double calculatePerimeter() {
return 2 * (width + height);
}
// 计算面积的方法
public double calculateArea() {
return width * height;
}
}
// 主程序部分
public class Main {
public static void main(String[] args) {
// 创建Rectangle对象
Rectangle rectangle = new Rectangle(5.0, 3.0);
// 计算并输出周长
System.out.println("The perimeter of the rectangle is: " + rectangle.calculatePerimeter());
// 计算并输出面积
System.out.println("The area of the rectangle is: " + rectangle.calculateArea());
}
}
```
在这个例子中,我们首先定义了一个Rectangle类,包含宽度和高度两个私有属性以及对应的构造函数。然后我们分别为计算周长和面积分别定义了calculatePerimeter和calculateArea方法。在主程序`main`方法里,我们创建了一个Rectangle对象,并通过它调用这两个方法来获取结果。
创建一个Rectangle类(矩形类),添加2个属性width、height,添加2个方法计算矩形的周长和面积。编程利用Rectangle类的对象计算并输出一个矩形的周长和面积。
好的,以下是创建Rectangle类并计算周长和面积的Python代码:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def perimeter(self):
return 2 * (self.width + self.height)
def area(self):
return self.width * self.height
# 创建一个矩形对象
rect = Rectangle(5, 10)
# 计算并输出矩形的周长和面积
print("矩形的周长为:", rect.perimeter())
print("矩形的面积为:", rect.area())
```
输出结果为:
```
矩形的周长为: 30
矩形的面积为: 50
```
其中,`__init__`方法用于初始化对象的属性,`perimeter`方法用于计算矩形的周长,`area`方法用于计算矩形的面积。我们创建了一个矩形对象`rect`,并调用其`perimeter`和`area`方法计算矩形的周长和面积,最后输出结果。
阅读全文
相关推荐
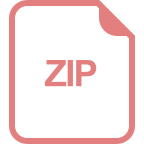
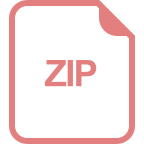
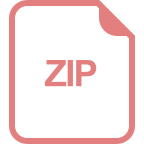














