c++ 高精度浮点书
时间: 2023-11-18 19:51:48 浏览: 131
对于高精度浮点数的处理,可以使用任意精度的浮点数库来实现。常见的库有GMP(GNU Multiple Precision Arithmetic Library)、MPFR(Multiple Precision Floating-Point Reliable)、BigDecimal等。这些库可以提供更高的精度和更强的精确性。
相关问题
c++高精度减法浮点型
对于浮点数的高精度减法,可以将浮点数转化为字符串,然后按照高精度减法的思路进行计算,最后再将结果转化为浮点数。
以下是一个使用字符串实现高精度减法的例子:
```c++
#include <iostream>
#include <string>
#include <algorithm> // for reverse()
using namespace std;
string float_sub(string a, string b) {
int lena = a.length(), lenb = b.length();
int dotPosa = a.find('.'), dotPosb = b.find('.');
int lenDeca = (dotPosa == -1 ? 0 : lena - dotPosa - 1);
int lenDecb = (dotPosb == -1 ? 0 : lenb - dotPosb - 1);
// 补足小数点后的0
if (lenDeca < lenDecb) {
a.append(lenDecb - lenDeca, '0');
} else if (lenDecb < lenDeca) {
b.append(lenDeca - lenDecb, '0');
}
// 补足小数点前的0
if (dotPosa < dotPosb) {
a.insert(0, dotPosb - dotPosa, '0');
} else if (dotPosb < dotPosa) {
b.insert(0, dotPosa - dotPosb, '0');
}
// 对齐后的长度
int len = max(lena, lenb);
// 去掉小数点
a.erase(dotPosa, 1);
b.erase(dotPosb, 1);
// 翻转字符串方便计算
reverse(a.begin(), a.end());
reverse(b.begin(), b.end());
// 计算
string c(len, '0');
int carry = 0;
for (int i = 0; i < len; i++) {
int numa = (i < lena ? a[i] - '0' : 0);
int numb = (i < lenb ? b[i] - '0' : 0);
int numc = numa - numb - carry;
if (numc < 0) {
numc += 10;
carry = 1;
} else {
carry = 0;
}
c[i] = numc + '0';
}
// 去掉前导0
while (c.length() > 1 && c.back() == '0') {
c.pop_back();
}
// 加上小数点
int lenDec = max(lenDeca, lenDecb);
if (lenDec > 0) {
c.insert(len - lenDec, ".");
}
// 翻转回来
reverse(c.begin(), c.end());
return c;
}
int main() {
string a = "123.456";
string b = "78.9";
string c = float_sub(a, b);
cout << c << endl;
return 0;
}
```
这个例子中,我们首先将小数点后的0补足,然后将小数点前的0也补足,接着去掉小数点,翻转字符串,进行高精度减法计算,再去掉前导0,加上小数点,最后翻转回来,得到最终结果。
c++高精度减法浮点型 不用函数
不使用函数的高精度浮点数减法可以按照以下步骤进行:
1. 将浮点数转化为字符串形式
2. 找到小数点位置,并计算出小数点前和小数点后的位数
3. 分别补足小数点前和小数点后的0,使两个浮点数的小数点前和小数点后的位数相等
4. 去掉小数点
5. 从字符串的最后一位(也就是从小数点后面的数字开始)开始,按照高精度减法的方法计算两个数的差,注意进位和借位
6. 处理结果,加上小数点,去掉前导0,翻转字符串,转化为浮点数
代码实现如下:
```c++
#include <iostream>
#include <string>
#include <algorithm> // for reverse()
using namespace std;
int main() {
string a = "123.456";
string b = "78.9";
// 找到小数点位置
int dotPosa = a.find('.');
int dotPosb = b.find('.');
// 计算小数点前和小数点后的位数
int lenDeca = (dotPosa == -1 ? 0 : a.length() - dotPosa - 1);
int lenDecb = (dotPosb == -1 ? 0 : b.length() - dotPosb - 1);
int lenInta = (dotPosa == -1 ? a.length() : dotPosa);
int lenIntb = (dotPosb == -1 ? b.length() : dotPosb);
// 补足小数点前的0
if (lenInta < lenIntb) {
a.insert(0, lenIntb - lenInta, '0');
} else if (lenIntb < lenInta) {
b.insert(0, lenInta - lenIntb, '0');
}
// 补足小数点后的0
if (lenDeca < lenDecb) {
a.append(lenDecb - lenDeca, '0');
} else if (lenDecb < lenDeca) {
b.append(lenDeca - lenDecb, '0');
}
// 去掉小数点
a.erase(dotPosa, 1);
b.erase(dotPosb, 1);
// 翻转字符串
reverse(a.begin(), a.end());
reverse(b.begin(), b.end());
// 高精度减法
string c = "";
int carry = 0;
for (int i = 0; i < a.length(); i++) {
int numa = a[i] - '0';
int numb = (i < b.length() ? b[i] - '0' : 0);
int numc = numa - numb - carry;
if (numc < 0) {
numc += 10;
carry = 1;
} else {
carry = 0;
}
c += (char)(numc + '0');
}
// 去掉前导0
while (c.length() > 1 && c.back() == '0') {
c.pop_back();
}
// 加上小数点
if (lenDeca > 0) {
c.insert(lenDeca, ".");
}
// 翻转回来
reverse(c.begin(), c.end());
// 输出结果
cout << c << endl;
return 0;
}
```
注意,在这个例子中,我们没有考虑负数的情况。如果需要处理负数,可以在高精度减法的过程中,先判断两个数的大小关系,再决定是否需要加上负号。
阅读全文
相关推荐
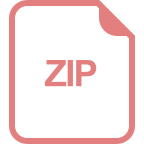
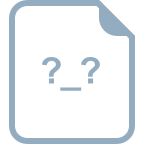
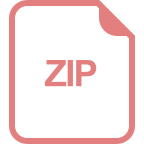
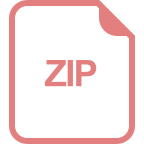
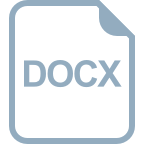
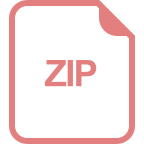
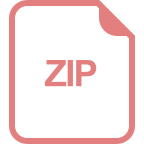
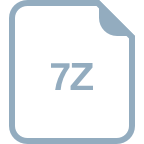
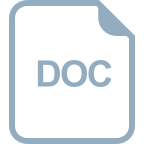
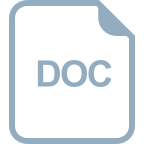
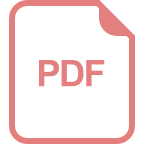
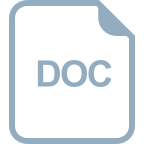
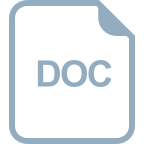
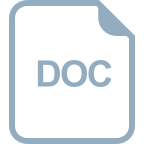