使用MongoDB Driver做多字段查询时,还有哪些查询运算符可供选择?
时间: 2024-09-27 21:07:31 浏览: 23
在使用MongoDB Driver进行多字段查询时,除了基本的比较运算符(如`$eq`, `$gt`, `$lt`, `$gte`, `$lte`),还有一些更复杂的运算符供选择:
1. `$not`: 对特定条件取反,即查找不符合给定条件的文档。
2. `$in`: 匹配指定值列表中的任何一个字段值。
3. `$nin`: 匹配不在指定值列表中的字段值。
4. `$all`: 检查数组字段是否都包含给定的所有元素。
5. `$exists`: 确认字段是否存在,或者检查其值是否非空。
6. `$elemMatch`: 在嵌套数组中查找满足特定条件的元素。
7. `$type`: 检查字段的类型。
8. `$regex`: 正则表达式匹配,可以进行模糊搜索。
比如,如果你想查询名字在"John"和"Jane"之间的用户,可以使用`$in`:
```csharp
filter = Builders<BsonDocument>.Filter.In("Name", new BsonArray(new string[] { "John", "Jane" }));
```
每个运算符都有相应的构建器方法,如`Builders<BsonDocument>.Filter.In`,可以根据需要选择使用。
相关问题
C# 连接mongodb多字段查询
在C#中连接MongoDB并进行多字段查询,通常使用MongoDB的官方驱动程序MongoDB.Driver,它提供了一个强大的`IMongoCollection<T>`接口来进行数据操作。假设我们有一个包含两个字段如`Name`和`Age`的文档集合,你可以通过`$and`、`$or`等查询运算符来进行多字段查询。
例如,如果你想找到所有名字为"John"并且年龄大于30的用户,可以这样做:
```csharp
using MongoDB.Bson;
using MongoDB.Driver;
var filter = Builders<BsonDocument>.Filter.And(
Builders<BsonDocument>.Filter.Eq("Name", "John"),
Builders<BsonDocument>.Filter.Gt("Age", 30)
);
IMongoCollection<BsonDocument> collection = yourMongoDatabase.GetCollection<BsonDocument>("yourCollectionName");
var results = await collection.Find(filter).ToListAsync();
```
这里,`Builders<BsonDocument>.Filter.Eq`用于等于比较,`Builders<BsonDocument>.Filter.Gt`用于大于比较。`$and`运算符将这两个条件组合起来。
mongodb 比较两个字段时间大小 java代码
在MongoDB中比较两个日期字段的时间大小,通常需要将日期转换成可以进行比较的格式,然后在查询操作中使用适当的条件。如果你正在使用Java驱动程序如`mongodb-java-driver`,你可以这样做:
```java
import org.bson.Document;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
// 假设你已经连接到MongoDB数据库并获取了对应的集合
MongoDatabase database = yourMongoDatabase;
MongoCollection<Document> collection = database.getCollection("your_collection");
// 定义查询文档,其中包含你要比较的日期字段
Document query = new Document();
query.append("dateField1", new Document("$gte", "2022-01-01T00:00:00Z")); // 比较大于等于这个日期
query.append("dateField2", new Document("$lt", "2023-01-01T00:00:00Z")); // 比较小于这个日期
// 执行查询
MongoCursor<Document> cursor = collection.find(query).iterator();
while (cursor.hasNext()) {
Document document = cursor.next();
// 获取到满足条件的文档
}
// 关闭游标
cursor.close();
```
这里假设`dateField1`和`dateField2`是你想要比较的日期字段名。`$gte`和`$lt`分别是MongoDB的查询运算符,分别代表"greater than or equal to"和"less than"。
阅读全文
相关推荐
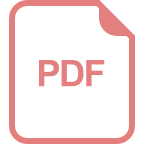
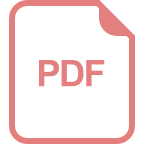
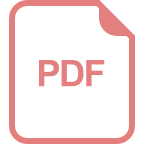
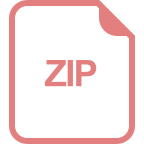
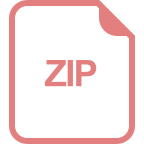
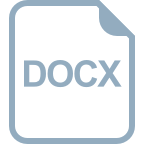
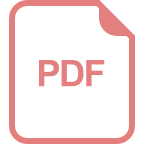
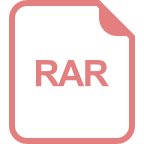
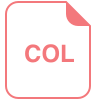
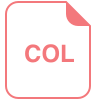

