C++用队列实现学生在线答疑系统
时间: 2024-10-25 18:13:06 浏览: 22
在C++中,可以使用`std::queue`容器来实现学生在线答疑系统的简单模型。`std::queue`是一种先进先出(First-In-First-Out,FIFO)的数据结构,非常适合处理一系列请求,如学生的提问。
首先,你可以创建一个数据结构来表示学生或问题,比如`Question`类:
```cpp
class Question {
public:
string studentName;
string question;
// 其他可能的属性...
};
```
然后定义一个队列来存储这些问题:
```cpp
#include <queue>
using namespace std;
vector<Question> queueOfQuestions;
priority_queue<Question> unansweredQueue; // 如果你想优先处理未解答的问题
```
接下来,当你有新的问题加入时,可以这样做:
```cpp
void addQuestion(string studentName, string question) {
Question newQuestion = {studentName, question};
queueOfQuestions.push_back(newQuestion);
unansweredQueue.push(newQuestion); // 如果需要优先级,这里放进去
}
```
对于教师来说,可以从队列中取出并处理问题:
```cpp
void answerNextQuestion() {
if (!unansweredQueue.empty()) {
Question currentQuestion = unansweredQueue.top();
// 这里处理问题,例如添加答案到数据库或通知
unansweredQueue.pop(); // 解答完移除
} else if (!queueOfQuestions.empty()) {
// 如果所有未解答的都已处理,从普通队列取下一个
currentQuestion = queueOfQuestions.front();
queueOfQuestions.pop();
// 同样在这里处理问题...
}
}
```
这只是一个基础的例子,实际系统可能会更复杂,包括并发控制、错误处理以及网络通信等。
阅读全文
相关推荐
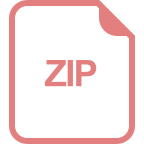
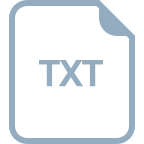
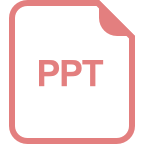
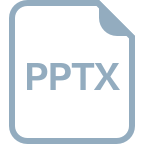
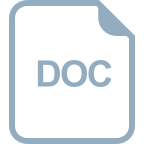
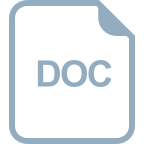
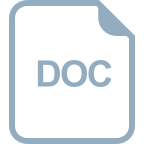
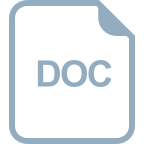
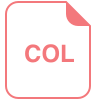
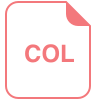
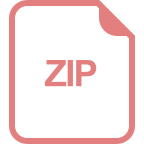
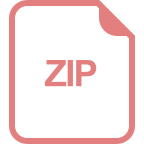
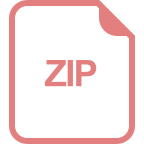
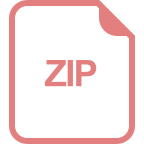
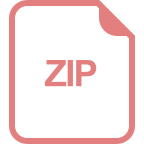