编写C语言函数求3!+6!+9!+12!+15!+18!+21!
时间: 2024-11-20 15:27:43 浏览: 6
编写C语言函数来计算3! + 6! + 9! + 12! + 15! + 18! + 21! 的过程可以分解为几个步骤:
1. 定义一个递归函数来计算阶乘,因为阶乘通常涉及到较大的数和递归关系。
2. 创建一个主函数,用于调用这个阶乘函数,并累加结果。
以下是示例代码:
```c
#include <stdio.h>
// 阶乘函数,使用递归实现
unsigned long long factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
// 主函数,累加阶乘的结果
int main() {
unsigned long long result = 0;
// 计算并累加各个阶乘
for (int i = 3; i <= 21; i += 3) {
result += factorial(i);
}
printf("3! + 6! + 9! + 12! + 15! + 18! + 21! = %llu\n", result);
return 0;
}
```
运行此程序将计算并显示给定阶乘之和。请注意,在C语言中,`unsigned long long`被用于存储大整数,因为计算过程中可能会得到很大的数值。
相关问题
c语言程序设计编写函数求3!+6!+9!+12!+15!+18!+21!
根据引用[1],我们可以编写一个函数来计算1/2!+1/4!+…+1/m!的值,然后根据题目要求,将3、6、9、12、15、18、21分别代入函数中计算,最后将结果相加即可得到答案。以下是C语言程序设计编写函数求3!+6!+9!+12!+15!+18!+21!的代码实现:
```c
#include <stdio.h>
double fun(int m) {
double sum = 0;
double temp = 1;
for (int i = 2; i <= m; i += 2) {
temp *= (i - 1) * i;
sum += 1 / temp;
}
return sum;
}
int main() {
int sum = 0;
for (int i = 3; i <= 21; i += 3) {
sum += fun(i);
}
printf("3!+6!+9!+12!+15!+18!+21!=%d\n", sum);
return 0;
}
```
编写C语言程序求2!+ 4! +6!+ 8!+ 10! +12!
编写C语言程序求阶乘之和,我们需要定义一个递归函数来计算阶乘,并遍历给定数列求和。这里是一个简单的示例:
```c
#include <stdio.h>
// 定义阶乘函数
unsigned long long factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
int main() {
int numbers[] = {2, 4, 6, 8, 10, 12}; // 要相加的阶乘数
int size = sizeof(numbers) / sizeof(numbers[0]);
unsigned long long result = 0;
for (int i = 0; i < size; i++) {
result += factorial(numbers[i]);
}
printf("2! + 4! + 6! + 8! + 10! + 12! = %llu\n", result);
return 0;
}
```
这个程序首先定义了一个`factorial`函数来计算阶乘,然后在主函数中通过循环将各个数的阶乘累加到`result`变量中。最后输出总和。
阅读全文
相关推荐
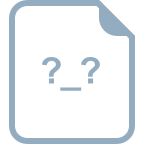
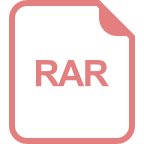













